Student student3 = new Student( name: "1", age: 2); Student student1 = new Student( name: "1", age: 2); Student student2 = new Student( name: "1", age: 2); List<Student> strList = new ArrayList<>(); strList.add(student3); strList.add(student1); strList.add(student2); System.out.println("name:1"+"age:2,2,2") 请问下这个list要输出我打印的那个 逻辑要怎么写
时间: 2024-01-30 09:03:49 浏览: 20
你可以按以下方式遍历 list,并且在输出每个元素之前检查其属性是否与期望值匹配:
```
for (Student student : strList) {
if (student.getName().equals("1") && student.getAge() == 2) {
System.out.println("name:1, age:2");
}
}
```
这将遍历 list 中的每个学生对象,检查其姓名和年龄是否与预期匹配,如果匹配,则输出 "name:1, age:2"。如果不匹配,则不会输出任何内容。
相关问题
定义下面三个字典,请将其存储在列表中,并遍历输出每一个字典信息。 student_info1 = {'name':'Wangmi', 'sex':'F', 'age':'15'} student_info2 = {'name':'Linmei', 'sex':'M', 'age':'14'} student_info3 = {'name':'Chenhui', 'sex':'F', 'age':'14'}
以下是您所需的代码:
```python
# 定义三个字典
student_info1 = {'name':'Wangmi', 'sex':'F', 'age':'15'}
student_info2 = {'name':'Linmei', 'sex':'M', 'age':'14'}
student_info3 = {'name':'Chenhui', 'sex':'F', 'age':'14'}
# 将三个字典存储在列表中
student_list = [student_info1, student_info2, student_info3]
# 遍历列表并输出每个字典的信息
for student in student_list:
print("Name: " + student['name'])
print("Sex: " + student['sex'])
print("Age: " + student['age'])
print("---------------")
```
输出结果如下:
```
Name: Wangmi
Sex: F
Age: 15
---------------
Name: Linmei
Sex: M
Age: 14
---------------
Name: Chenhui
Sex: F
Age: 14
---------------
```
def sort(): find_all(name_list) if os.path.exists('students.txt'): with open('students.txt', 'r', encoding='utf-8') as rfile: students = rfile.readlines() student_new = [] for item in students: d = eval(item) student_new.append(d) else: return asc_or_desc = input('请选择排序方式(0,升序 1,降序)\n') if asc_or_desc == '0': asc_or_desc_bool = False elif asc_or_desc == '1': asc_or_desc_bool = True else: print('您的输入有误,请重新输入') sort() mode = input('请选择排序方式(1,按年龄排序 2,按英语成绩排序 3,按Python成绩排序 4,按Java成绩排序 5,按Mysql成绩排序)\n') if mode == '1': student_new.sort(key=lambda student_new: int(student_new[0]['age']), reverse=asc_or_desc_bool) elif mode == '2': student_new.sort(key=lambda student_new: int(student_new[0]['English']), reverse=asc_or_desc_bool) elif mode == '3': student_new.sort(key=lambda student_new: int(student_new[0]['Python']), reverse=asc_or_desc_bool) elif mode == '4': student_new.sort(key=lambda student_new: int(student_new[0]['Java']), reverse=asc_or_desc_bool) elif mode == '5': student_new.sort(key=lambda student_new: int(student_new[0]['Mysql']), reverse=asc_or_desc_bool) else: print('您输入的信息有误,请重新输入') sort() find_all(student_new)
这段代码中的 `find_all()` 函数没有被定义,需要在代码中定义或者调用正确的函数名。此外,在调用 `find_all()` 函数时,需要传入一个参数,即 `name_list`。因此,你需要修改代码,使其正确定义 `find_all()` 函数并传入正确的参数。
另外,如果用户输入的排序方式不正确,代码应该提示用户重新输入,并重新调用 `sort()` 函数,而不是直接退出。
下面是修改后的代码:
```
import os
def find_all(name_list):
# 定义或调用正确的函数
def sort(name_list):
find_all(name_list)
if os.path.exists('students.txt'):
with open('students.txt', 'r', encoding='utf-8') as rfile:
students = rfile.readlines()
student_new = []
for item in students:
d = eval(item)
student_new.append(d)
else:
return
asc_or_desc = input('请选择排序方式(0,升序 1,降序)\n')
if asc_or_desc == '0':
asc_or_desc_bool = False
elif asc_or_desc == '1':
asc_or_desc_bool = True
else:
print('您的输入有误,请重新输入')
sort(name_list)
mode = input('请选择排序方式(1,按年龄排序 2,按英语成绩排序 3,按Python成绩排序 4,按Java成绩排序 5,按Mysql成绩排序)\n')
if mode == '1':
student_new.sort(key=lambda student_new: int(student_new[0]['age']), reverse=asc_or_desc_bool)
elif mode == '2':
student_new.sort(key=lambda student_new: int(student_new[0]['English']), reverse=asc_or_desc_bool)
elif mode == '3':
student_new.sort(key=lambda student_new: int(student_new[0]['Python']), reverse=asc_or_desc_bool)
elif mode == '4':
student_new.sort(key=lambda student_new: int(student_new[0]['Java']), reverse=asc_or_desc_bool)
elif mode == '5':
student_new.sort(key=lambda student_new: int(student_new[0]['Mysql']), reverse=asc_or_desc_bool)
else:
print('您输入的信息有误,请重新输入')
sort(name_list)
find_all(student_new)
```
注意,这里只是修改了 `sort()` 函数中的部分代码,而其他部分的代码可能还需要进行调整或修改。
相关推荐
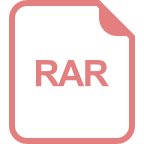












