python实现1。先按先序遍历序列建立二叉树的二叉链表; 2.用递归方式实现以下操作: 1)计算二叉树结点总数 2)计算二叉树深度 3)计算二叉树叶子结点总数 4)交换二叉树每个结点的左孩子和右孩子。
时间: 2024-05-09 13:17:18 浏览: 65
好的,我知道了。对于你的问题,我可以给你以下 Python 代码实现:
``` python
# 定义二叉树节点
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
# 按先序遍历序列建立二叉树的二叉链表
def buildTree(preorder):
if not preorder:
return None
root = TreeNode(preorder[0])
idx = 1
while idx < len(preorder) and preorder[idx] <= preorder[0]:
idx += 1
root.left = buildTree(preorder[1:idx])
root.right = buildTree(preorder[idx:])
return root
# 计算二叉树结点总数
def countNodes(root):
if not root:
return 0
return 1 + countNodes(root.left) + countNodes(root.right)
# 计算二叉树深度
def maxDepth(root):
if not root:
return 0
return 1 + max(maxDepth(root.left), maxDepth(root.right))
# 计算二叉树叶子结点总数
def countLeaves(root):
if not root:
return 0
if not root.left and not root.right:
return 1
return countLeaves(root.left) + countLeaves(root.right)
# 交换二叉树每个结点的左孩子和右孩子
def swapNodes(root):
if not root:
return
root.left, root.right = root.right, root.left
swapNodes(root.left)
swapNodes(root.right)
```
希望这个代码可以解决你的问题。如果你有其他问题,随时问我哦。
阅读全文
相关推荐
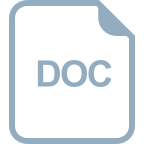
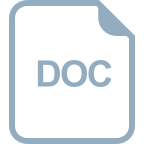
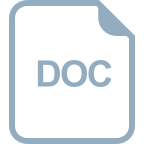












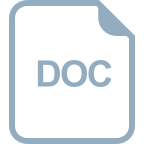
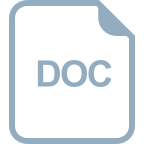