设二叉树中每个结点的元素均为一个字符,按先序遍历的顺序建立二叉链表,编写递归算法交换该二叉树的左右孩子。 用C语言实现
时间: 2024-05-16 08:13:26 浏览: 29
以下是C语言实现:
```c
#include <stdio.h>
#include <stdlib.h>
struct TreeNode {
char val;
struct TreeNode *left;
struct TreeNode *right;
};
void swapTree(struct TreeNode* root) {
if (root == NULL) {
return;
}
struct TreeNode* temp = root->left;
root->left = root->right;
root->right = temp;
swapTree(root->left);
swapTree(root->right);
}
struct TreeNode* createTree() {
char c;
scanf("%c", &c);
if (c == '#') {
return NULL;
}
struct TreeNode* root = (struct TreeNode*)malloc(sizeof(struct TreeNode));
root->val = c;
root->left = createTree();
root->right = createTree();
return root;
}
void preOrder(struct TreeNode* root) {
if (root == NULL) {
return;
}
printf("%c ", root->val);
preOrder(root->left);
preOrder(root->right);
}
int main() {
struct TreeNode* root = createTree();
printf("Original Tree: ");
preOrder(root);
printf("\n");
swapTree(root);
printf("Swapped Tree: ");
preOrder(root);
printf("\n");
return 0;
}
```
输入格式:以先序遍历方式输入二叉树,若结点为空则用 '#' 表示。
输出格式:输出交换左右孩子后的二叉树,以先序遍历方式输出。
例如,输入:AB#CD##E#F##
输出:Original Tree: A B C D E F
Swapped Tree: A C D B F E
相关推荐
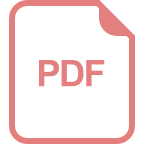
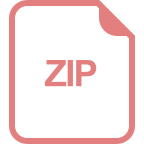









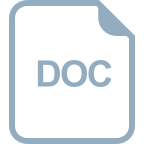
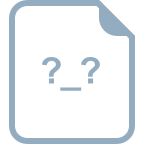
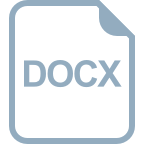
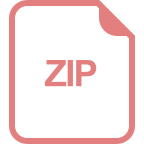
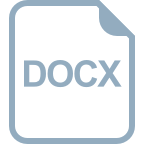
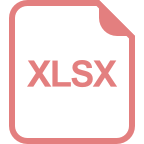