用devc++编写用折半查找法查找给定值a和b之间的所有元素(a<=b)。
时间: 2024-12-18 13:17:57 浏览: 0
在Dev-C++中,你可以使用折半查找法(也称为二分查找法)来在一个已排序的数组中查找特定范围内的所有元素。以下是实现这一功能的基本步骤:
1. 定义一个函数`binarySearchRange(arr[], a, b, target)`, 其中`arr[]`是输入数组,`a`和`b`分别是范围的开始和结束索引,`target`是要查找的目标值。
```cpp
#include <iostream>
using namespace std;
// Binary search function to find the first occurrence of 'target'
int binarySearch(int arr[], int left, int right, int target) {
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == target)
return mid; // Return index if found
else if (arr[mid] < target)
left = mid + 1; // If target is greater than mid, search in right half
else
right = mid - 1; // If target is less than mid, search in left half
}
return -1; // Target not found, return -1
}
// Function to find all elements between a and b using binary search
void printElementsInRange(int arr[], int n, int a, int b, int target) {
int firstIndex = binarySearch(arr, a, b, target);
// If target is found within range
if (firstIndex != -1) {
// Print all occurrences of target from a to b
while (a <= b && arr[a] == target) {
cout << arr[a++] << " ";
}
// Search for next occurrence after a
firstIndex++;
for (int i = firstIndex; i <= b && arr[i] == target; i++) {
cout << arr[i] << " ";
}
} else {
cout << "Target not present in given range.\n";
}
}
```
2. 调用`printElementsInRange()`函数,并传入你的数组、数组长度以及查找的范围。
```cpp
int main() {
int arr[] = {1, 2, 2, 3, 4, 5, 6, 7, 7, 8, 9};
int n = sizeof(arr) / sizeof(arr[0]);
int a = 3, b = 8, target = 7;
printElementsInRange(arr, n, a, b, target);
return 0;
}
```
阅读全文
相关推荐
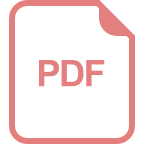
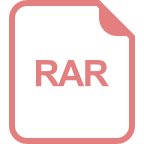















