可以帮我配合着easyx+c++语言写一个五子棋游戏么
时间: 2024-01-14 07:04:16 浏览: 85
当然可以!这里给您提供一个简单的五子棋游戏的代码示例,使用了 EasyX 图形库和 C++ 语言。
```c++
#include <graphics.h>
#include <conio.h>
#include <iostream>
using namespace std;
const int WIN_WIDTH = 640; // 窗口宽度
const int WIN_HEIGHT = 640; // 窗口高度
const int ROWS = 15; // 棋盘行数
const int COLS = 15; // 棋盘列数
const int BLOCK_SIZE = 40; // 棋盘格子大小
int board[ROWS][COLS] = {0}; // 棋盘状态,0表示空,1表示黑子,2表示白子
bool is_black_turn = true; // 是否轮到黑子
bool game_over = false; // 游戏是否结束
// 绘制棋盘
void draw_board() {
setbkcolor(WHITE);
cleardevice();
// 绘制横线
for (int i = 0; i < ROWS; i++) {
line(BLOCK_SIZE, BLOCK_SIZE * (i + 1), BLOCK_SIZE * COLS, BLOCK_SIZE * (i + 1));
}
// 绘制竖线
for (int i = 0; i < COLS; i++) {
line(BLOCK_SIZE * (i + 1), BLOCK_SIZE, BLOCK_SIZE * (i + 1), BLOCK_SIZE * ROWS);
}
// 绘制棋子
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
if (board[i][j] == 1) {
setfillcolor(BLACK);
solidcircle(BLOCK_SIZE * (j + 1), BLOCK_SIZE * (i + 1), BLOCK_SIZE / 2);
} else if (board[i][j] == 2) {
setfillcolor(WHITE);
solidcircle(BLOCK_SIZE * (j + 1), BLOCK_SIZE * (i + 1), BLOCK_SIZE / 2);
}
}
}
// 刷新屏幕
flushbatch();
}
// 判断是否胜利
bool check_win(int x, int y) {
// 水平方向
int count = 1;
for (int i = x - 1; i >= 0 && board[i][y] == board[x][y]; i--) count++;
for (int i = x + 1; i < ROWS && board[i][y] == board[x][y]; i++) count++;
if (count >= 5) return true;
// 垂直方向
count = 1;
for (int j = y - 1; j >= 0 && board[x][j] == board[x][y]; j--) count++;
for (int j = y + 1; j < COLS && board[x][j] == board[x][y]; j++) count++;
if (count >= 5) return true;
// 左上到右下方向
count = 1;
for (int i = x - 1, j = y - 1; i >= 0 && j >= 0 && board[i][j] == board[x][y]; i--, j--) count++;
for (int i = x + 1, j = y + 1; i < ROWS && j < COLS && board[i][j] == board[x][y]; i++, j++) count++;
if (count >= 5) return true;
// 右上到左下方向
count = 1;
for (int i = x - 1, j = y + 1; i >= 0 && j < COLS && board[i][j] == board[x][y]; i--, j++) count++;
for (int i = x + 1, j = y - 1; i < ROWS && j >= 0 && board[i][j] == board[x][y]; i++, j--) count++;
if (count >= 5) return true;
return false;
}
// 处理鼠标点击事件
void handle_mouse_click(int x, int y) {
if (game_over) return;
// 计算行列号
int row = y / BLOCK_SIZE - 1;
int col = x / BLOCK_SIZE - 1;
// 判断是否越界
if (row < 0 || row >= ROWS || col < 0 || col >= COLS) return;
// 判断是否已经有棋子
if (board[row][col] != 0) return;
// 下子
if (is_black_turn) {
board[row][col] = 1;
} else {
board[row][col] = 2;
}
// 判断是否胜利
if (check_win(row, col)) {
game_over = true;
if (is_black_turn) {
cout << "黑方胜利!" << endl;
} else {
cout << "白方胜利!" << endl;
}
}
// 切换下子方
is_black_turn = !is_black_turn;
// 重新绘制棋盘
draw_board();
}
int main() {
// 初始化窗口
initgraph(WIN_WIDTH, WIN_HEIGHT, SHOWCONSOLE);
// 绘制棋盘
draw_board();
// 处理鼠标点击事件
setbkcolor(WHITE);
setmousequeuestatus(MOUSE_NOWMOVING);
while (!game_over) {
MOUSEMSG m = GetMouseMsg();
if (m.uMsg == WM_LBUTTONDOWN) {
handle_mouse_click(m.x, m.y);
}
}
// 关闭窗口
closegraph();
return 0;
}
```
您可以将上述代码拷贝到 C++ 编辑器中编译运行,就可以玩五子棋游戏了。
阅读全文
相关推荐
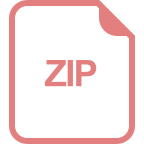
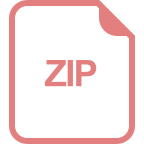


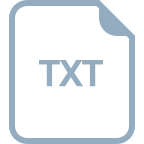
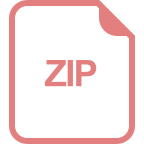
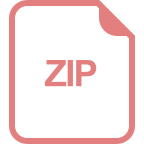
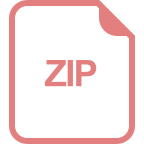
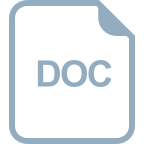
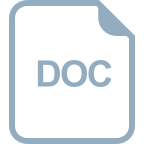
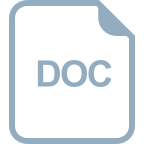
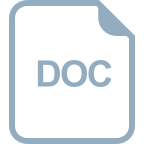
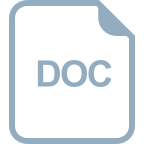

