vertices = all_vertices[self.face_ind, :]是什么意思
时间: 2024-02-29 16:57:22 浏览: 19
这是一个Python代码语句,其中包含以下元素:
- `all_vertices`:一个NumPy数组,包含所有的顶点坐标,每一行代表一个顶点的坐标。
- `self.face_ind`:一个索引数组,用来选择`all_vertices`中的特定行。这个索引数组可能是一个Python列表或NumPy数组,其中存储的是整数值,代表要选择的行的索引。
- `[:]`:这是Python中的切片操作符,用于选择数组中的一部分元素。在这里,它与索引数组一起使用,表示选择`all_vertices`数组中的特定行。
- `vertices`:这是一个新的NumPy数组,包含了从`all_vertices`中选择的特定行,即代表一个面(或多边形)的所有顶点坐标。
因此,该代码行的目的是从所有顶点的数组中选择与当前面相关的顶点,并将它们存储在一个新的数组中,以便后续处理。
相关问题
current_dir = os.path.dirname(os.path.realpath(__file__)) data_dir = os.path.join(current_dir, 'data') class Model(nn.Module): def __init__(self, template_path): super(Model, self).__init__() # set template mesh self.template_mesh = jr.Mesh.from_obj(template_path, dr_type='n3mr') self.vertices = (self.template_mesh.vertices * 0.5).stop_grad() self.faces = self.template_mesh.faces.stop_grad() self.textures = self.template_mesh.textures.stop_grad() # optimize for displacement map and center self.displace = jt.zeros(self.template_mesh.vertices.shape) self.center = jt.zeros((1, 1, 3)) # define Laplacian and flatten geometry constraints self.laplacian_loss = LaplacianLoss(self.vertices[0], self.faces[0]) self.flatten_loss = FlattenLoss(self.faces[0]) def execute(self, batch_size): base = jt.log(self.vertices.abs() / (1 - self.vertices.abs())) centroid = jt.tanh(self.center) vertices = (base + self.displace).sigmoid() * nn.sign(self.vertices) vertices = nn.relu(vertices) * (1 - centroid) - nn.relu(-vertices) * (centroid + 1) vertices = vertices + centroid # apply Laplacian and flatten geometry constraints laplacian_loss = self.laplacian_loss(vertices).mean() flatten_loss = self.flatten_loss(vertices).mean() return jr.Mesh(vertices.repeat(batch_size, 1, 1), self.faces.repeat(batch_size, 1, 1), dr_type='n3mr'), laplacian_loss, flatten_loss 在每行代码后添加注释
# 导入必要的包
import os
import jittor as jt
from jittor import nn
import jrender as jr
# 定义数据文件夹路径
current_dir = os.path.dirname(os.path.realpath(__file__))
data_dir = os.path.join(current_dir, 'data')
# 定义模型类
class Model(nn.Module):
def __init__(self, template_path):
super(Model, self).__init__()
# 设置模板网格
self.template_mesh = jr.Mesh.from_obj(template_path, dr_type='n3mr')
self.vertices = (self.template_mesh.vertices * 0.5).stop_grad() # 顶点坐标
self.faces = self.template_mesh.faces.stop_grad() # 面
self.textures = self.template_mesh.textures.stop_grad() # 纹理
# 优化位移贴图和中心点
self.displace = jt.zeros(self.template_mesh.vertices.shape) # 位移贴图
self.center = jt.zeros((1, 1, 3)) # 中心点坐标
# 定义拉普拉斯约束和平坦几何约束
self.laplacian_loss = LaplacianLoss(self.vertices[0], self.faces[0])
self.flatten_loss = FlattenLoss(self.faces[0])
def execute(self, batch_size):
base = jt.log(self.vertices.abs() / (1 - self.vertices.abs())) # 基础值
centroid = jt.tanh(self.center) # 中心点
vertices = (base + self.displace).sigmoid() * nn.sign(self.vertices) # 顶点坐标
vertices = nn.relu(vertices) * (1 - centroid) - nn.relu(-vertices) * (centroid + 1) # 顶点坐标变换
vertices = vertices + centroid # 顶点坐标变换
# 应用拉普拉斯约束和平坦几何约束
laplacian_loss = self.laplacian_loss(vertices).mean() # 拉普拉斯约束损失
flatten_loss = self.flatten_loss(vertices).mean() # 平坦几何约束损失
return jr.Mesh(vertices.repeat(batch_size, 1, 1), # 重复顶点坐标
self.faces.repeat(batch_size, 1, 1), # 重复面
dr_type='n3mr'), laplacian_loss, flatten_loss
def __init__(self, template_path): super(Model, self).__init__() # set template mesh self.template_mesh = jr.Mesh.from_obj(template_path, dr_type='n3mr') self.vertices = (self.template_mesh.vertices * 0.5).stop_grad() self.faces = self.template_mesh.faces.stop_grad() self.textures = self.template_mesh.textures.stop_grad() # optimize for displacement map and center self.displace = jt.zeros(self.template_mesh.vertices.shape) self.center = jt.zeros((1, 1, 3)) # define Laplacian and flatten geometry constraints self.laplacian_loss = LaplacianLoss(self.vertices[0], self.faces[0]) self.flatten_loss = FlattenLoss(self.faces[0])在每行代码后添加注释
```
def __init__(self, template_path):
super(Model, self).__init__() # 初始化父类
# 加载模板网格
self.template_mesh = jr.Mesh.from_obj(template_path, dr_type='n3mr')
# 缩放网格顶点坐标,并设置为不可求导
self.vertices = (self.template_mesh.vertices * 0.5).stop_grad()
# 设置网格面和纹理,并设置为不可求导
self.faces = self.template_mesh.faces.stop_grad()
self.textures = self.template_mesh.textures.stop_grad()
# 初始化位移和中心
self.displace = jt.zeros(self.template_mesh.vertices.shape) # 位移
self.center = jt.zeros((1, 1, 3)) # 中心点
# 定义 Laplacian 和 flatten 约束损失
self.laplacian_loss = LaplacianLoss(self.vertices[0], self.faces[0]) # Laplacian 约束损失
self.flatten_loss = FlattenLoss(self.faces[0]) # flatten 约束损失
```
注释解释如下:
- `super(Model, self).__init__()`:调用父类的构造函数进行初始化。
- `self.template_mesh = jr.Mesh.from_obj(template_path, dr_type='n3mr')`:从 OBJ 文件中加载模板网格。
- `self.vertices = (self.template_mesh.vertices * 0.5).stop_grad()`:将模板网格的顶点坐标缩放为原来的一半,并将其设置为不可求导。
- `self.faces = self.template_mesh.faces.stop_grad()`:将模板网格的面索引设置为不可求导。
- `self.textures = self.template_mesh.textures.stop_grad()`:将模板网格的纹理设置为不可求导。
- `self.displace = jt.zeros(self.template_mesh.vertices.shape)`:初始化位移变量,并将其设置为可求导的 0 张量。
- `self.center = jt.zeros((1, 1, 3))`:初始化中心点,并将其设置为可求导的 0 张量。
- `self.laplacian_loss = LaplacianLoss(self.vertices[0], self.faces[0])`:初始化 Laplacian 约束损失。
- `self.flatten_loss = FlattenLoss(self.faces[0])`:初始化 flatten 约束损失。
相关推荐
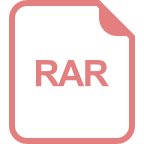
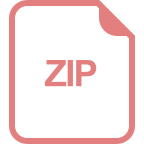
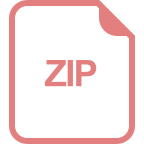
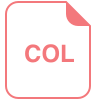
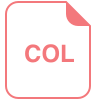
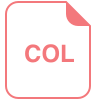
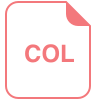









