二叉查找树的构建及序列化代码
时间: 2024-10-18 15:11:50 浏览: 39
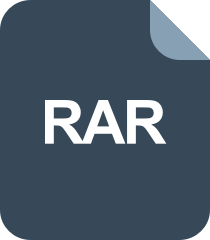
fininst.rar_audio matlab_audio simulink_fininst_matlab 二叉树_二叉查找树
二叉查找树(Binary Search Tree, BST),也称二叉搜索树,是一种特殊的二叉树,其中每个节点的左子树所有节点值都小于该节点,右子树所有节点值都大于该节点。构建BST的过程通常涉及插入新元素,而保证其性质。
**构建二叉查找树(插入操作)示例(Python):**
```python
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def insert_into_bst(root, val):
if not root:
return TreeNode(val)
elif val < root.val:
root.left = insert_into_bst(root.left, val)
else:
root.right = insert_into_bst(root.right, val)
return root
```
**序列化二叉查找树(转换成字符串)示例(Python):**
```python
def serialize_bst(root):
def helper(node, str_val):
if node is None:
return ''
left_str = helper(node.left, 'null')
right_str = helper(node.right, 'null')
return f'{str_val},{left_str},{right_str}'
return helper(root, str(root.val) if root else '')
# 反序列化
def deserialize_bst(serialized):
if serialized == 'null':
return None
values = serialized.split(',')
root = TreeNode(int(values[0]))
root.left = deserialize_bst(values[1])
root.right = deserialize_bst(values[2])
return root
```
阅读全文
相关推荐
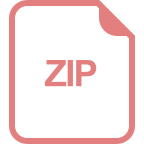
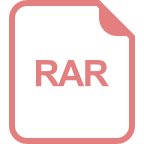



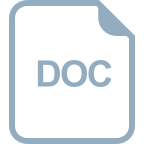
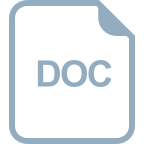
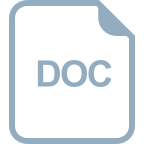
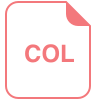
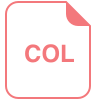
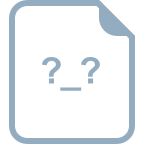
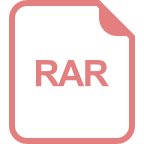
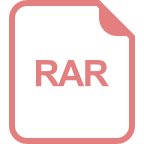
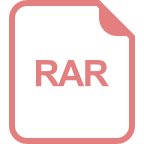
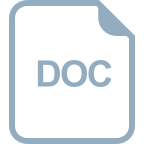
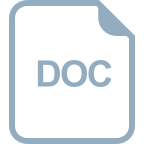
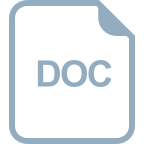
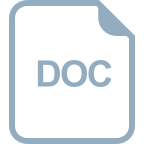