import org.junit.Test; import static org.junit.Assert.assertEquals; public class ErrorMessageTest { @Test public void testErrorMessageConstructor() { String code = "500"; Throwable throwable = new Throwable("Error occurred"); HttpServletRequest request = new MockHttpServletRequest(); HttpServletResponse response = new MockHttpServletResponse(); ErrorMessage errorMessage = new ErrorMessage(code, throwable, request, response); // Assert that the code is set correctly assertEquals(code, errorMessage.getCode()); } }这样会报红,java.lang.Throwable
时间: 2024-04-15 15:26:45 浏览: 17
看起来你在单元测试代码中仍然遇到了 "java.lang.Throwable" 异常。这可能是因为在你的代码中没有正确导入所需的类或接口。
请确保你已经正确导入了以下类和接口:
```java
import org.junit.Test;
import static org.junit.Assert.assertEquals;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
```
另外,为了解决 "java.lang.Throwable" 异常,请检查 `MockHttpServletRequest` 和 `MockHttpServletResponse` 类的导入情况。确保你使用的是正确的模拟类,并且它们正确实现了所需的接口。
如果你使用的是某个测试框架提供的模拟对象,例如 Spring Framework 提供的 `MockHttpServletRequest` 和 `MockHttpServletResponse`,请确保你已经正确添加了相应的依赖。
如果你自己实现了这些模拟类,确保它们正确地实现了相关接口和方法。如果你仍然无法解决问题,请提供更多关于 `MockHttpServletRequest` 和 `MockHttpServletResponse` 类的信息,以便我能够更好地帮助你排查问题。
相关问题
import static org.junit.Assert.assertEquals;
As an AI language model, I do not have the capability to execute code. However, I can provide an explanation of the code snippet you provided.
The `import` statement at the beginning of the code imports the `assertEquals` method from the `org.junit.Assert` class. This method is commonly used in Java unit testing to compare expected values with actual values.
The `static` keyword indicates that the method can be accessed without creating an instance of the class it belongs to. This means that you can use the `assertEquals` method directly in your code without creating an instance of the `Assert` class.
The `assertEquals` method takes two parameters: the expected value and the actual value. It checks if the two values are equal and throws an assertion error if they are not.
org.junit.jupiter.api.Test 和 org.junit.Test 差别
org.junit.jupiter.api.Test和org.junit.Test是JUnit测试框架中的两个不同的注解。它们之间的主要区别在于它们所在的JUnit版本。org.junit.jupiter.api.Test是JUnit 5中的注解,而org.junit.Test是JUnit 4中的注解。JUnit 5是JUnit测试框架的最新版本,它引入了许多新的功能和改进,包括对Java 8的支持,扩展模型,新的注解等等。因此,如果你使用JUnit 5,应该使用org.junit.jupiter.api.Test注解来编写测试用例。如果你使用JUnit 4,则应该使用org.junit.Test注解。
举个例子,如果你想在JUnit 5中编写一个简单的测试用例,可以这样写:
```java
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class MyTest {
@Test
public void test() {
String str = "JUnit 5 is better than JUnit 4";
assertEquals("JUnit 5 is better than JUnit 4", str);
}
}
```
如果你想在JUnit 4中编写相同的测试用例,可以这样写:
```java
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class MyTest {
@Test
public void test() {
String str = "JUnit 5 is better than JUnit 4";
assertEquals("JUnit 5 is better than JUnit 4", str);
}
}
```
相关推荐
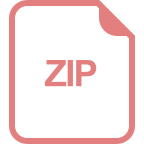
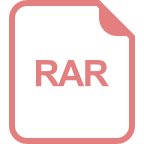












