1定义一个点,包含x,y坐标。包含无参构造函数和有参构造函数。 2定义一条边,拥有起点、终点,拥有长度属性(长度可计算) 3定义一个长方形,由四条边(或四个顶点)组成,拥有面积、周长属性(可计算)。判断其有效性。 4分别测试点对象、边对象和长方形对象。 用Java编写
时间: 2024-09-28 19:09:13 浏览: 29
在Java中,我们可以这样定义这三个类:
1. 点类 (Point):
```java
public class Point {
private double x;
private double y;
// 无参构造函数
public Point() {
this.x = 0;
this.y = 0;
}
// 有参构造函数
public Point(double x, double y) {
this.x = x;
this.y = y;
}
// 获取和设置坐标的方法
public double getX() { return x; }
public void setX(double newX) { x = newX; }
public double getY() { return y; }
public void setY(double newY) { y = newY; }
// 测试
public static void main(String[] args) {
Point p1 = new Point(); // 使用无参构造
Point p2 = new Point(1, 2); // 使用有参构造
}
}
```
2. 边类 (Edge):
```java
public class Edge {
private Point start;
private Point end;
private double length;
// 构造函数和getter/setter省略了
// 计算长度的方法
public double getLength() {
return Math.sqrt(Math.pow(end.getX() - start.getX(), 2) + Math.pow(end.getY() - start.getY(), 2));
}
// 测试
public static void main(String[] args) {
Edge e = new Edge(new Point(0, 0), new Point(3, 4)); // 定义边并计算长度
}
}
```
3. 长方形类 (Rectangle):
```java
import java.util.ArrayList;
public class Rectangle {
ArrayList<Point> vertices; // 四个顶点
private double width;
private double height;
// 构造函数、getter/setter以及计算面积和周长的方法省略了
// 判断有效性(例如,检查是否所有角点形成正方形)
public boolean isValid() {
if (vertices.size() != 4) return false;
for (int i = 0; i < 3; i++) {
if (!vertices.get(i).equals(vertices.get((i+1)%4))) return false;
}
// 其他条件判断...
return true;
}
// 测试
public static void main(String[] args) {
Rectangle rect = new Rectangle(new Point(0, 0), new Point(5, 0), new Point(5, 6), new Point(0, 6)); // 创建长方形实例并判断有效
}
}
```
相关推荐
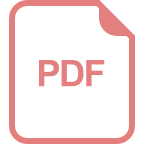
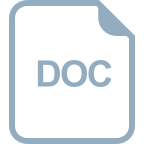
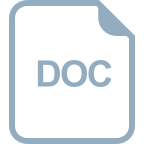















