1 定义—个点,包含X,y坐标。包含无参构造函数和有参构遥的数。 2 定义一条边,拥有起点、终点,拥有长魔属性(长度可计算) 3定义一个长方形,由四条边(或四个顶点)组成,拥有面积、周长属性(可计算)。判断其有效性。 4分别测试点对象、边对象和长方形对象。
时间: 2024-09-28 21:09:45 浏览: 37
1. 点类 (Point) 的定义通常是这样的:
```java
public class Point {
private double x;
private double y;
// 无参构造函数
public Point() {
this.x = 0.0;
this.y = 0.0;
}
// 有参构造函数
public Point(double x, double y) {
this.x = x;
this.y = y;
}
// getter and setter methods for x and y
public double getX() { return x; }
public void setX(double x) { this.x = x; }
public double getY() { return y; }
public void setY(double y) { this.y = y; }
}
```
2. 边类 (LineSegment):
```java
public class LineSegment {
private Point start;
private Point end;
private double length;
public LineSegment(Point start, Point end) {
this.start = start;
this.end = end;
this.length = calculateLength();
}
// 计算两点之间的距离
private double calculateLength() {
return Math.sqrt(Math.pow(end.getX() - start.getX(), 2) + Math.pow(end.getY() - start.getY(), 2));
}
// getter methods
public Point getStart() { return start; }
public Point getEnd() { return end; }
public double getLength() { return length; }
}
```
3. 长方形类 (Rectangle):
```java
public class Rectangle {
private Point topLeft;
private Point bottomRight;
private double width;
private double height;
public Rectangle(Point topLeft, Point bottomRight) {
this.topLeft = topLeft;
this.bottomRight = bottomRight;
this.width = bottomRight.getX() - topLeft.getX();
this.height = bottomRight.getY() - topLeft.getY();
checkValidity();
}
// 检查长宽是否为正数
private void checkValidity() {
if (width <= 0 || height <= 0) {
throw new IllegalArgumentException("Invalid rectangle dimensions");
}
}
// getters and setters for width and height
// ... (类似 Point 类的方法)
}
// 判断有效性通常在构造函数内部完成,如上面所示
```
4. 测试示例:
```java
public static void main(String[] args) {
Point p1 = new Point(1, 2);
Point p2 = new Point(4, 6);
LineSegment line = new LineSegment(p1, p2);
System.out.println("Line segment from (" + p1.getX() + ", " + p1.getY() + ") to (" + p2.getX() + ", " + p2.getY() + ") has length: " + line.getLength());
Rectangle rect = new Rectangle(new Point(0, 0), new Point(5, 10));
System.out.println("Rectangle area: " + rect.getArea());
System.out.println("Rectangle perimeter: " + rect.getCircumference());
}
```
阅读全文
相关推荐
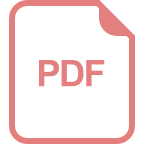
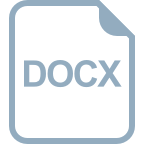
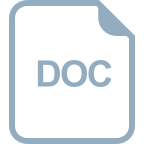















