用python写一段运算两个数点乘和叉乘的运算
时间: 2024-05-05 15:16:39 浏览: 89
点乘运算:
```python
a = [1, 2, 3]
b = [4, 5, 6]
dot_product = sum([a[i]*b[i] for i in range(len(a))])
print("Dot product: ", dot_product)
```
输出:
```
Dot product: 32
```
叉乘运算:
```python
a = [1, 2, 3]
b = [4, 5, 6]
cross_product = [a[1]*b[2]-a[2]*b[1], a[2]*b[0]-a[0]*b[2], a[0]*b[1]-a[1]*b[0]]
print("Cross product: ", cross_product)
```
输出:
```
Cross product: [-3, 6, -3]
```
相关问题
python点乘和叉乘
Python中的点乘和叉乘是两种不同的运算。
点乘是指对应元素相乘,即将两个数组或矩阵的对应位置的元素相乘。在Python中,可以使用`*`符号进行点乘运算。例如,对于两个数组A和B,可以使用`C = A * B`来进行点乘运算。点乘的结果是一个新的数组,其每个元素都是原数组对应位置元素的乘积。
叉乘是指矩阵的乘法运算。在Python中,可以使用`numpy`库的`dot`函数或`@`符号来进行矩阵的乘法运算。例如,对于两个矩阵A和B,可以使用`C = numpy.dot(A, B)`或`C = A @ B`来进行叉乘运算。叉乘的结果是一个新的矩阵,其维度满足矩阵乘法的要求。
需要注意的是,点乘和叉乘的操作对象不同,点乘是对应元素相乘,而叉乘是矩阵的乘法运算。因此,点乘的操作对象可以是数组,而叉乘的操作对象必须是矩阵。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *3* [Python学习-乘法操作:点乘和叉乘](https://blog.csdn.net/lj2048/article/details/113825968)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
- *2* [python数组(矩阵)乘法(点乘、叉乘)](https://blog.csdn.net/qq_32649321/article/details/123012420)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
设计一个三维向量类Vector3D,包含三维向量的一些基本运算,如加法、减法以及点乘、叉乘。
好的,下面是一个简单的 Vector3D 类的示例代码:
```python
class Vector3D:
def __init__(self, x, y, z):
self.x = x
self.y = y
self.z = z
def __add__(self, other):
return Vector3D(self.x + other.x, self.y + other.y, self.z + other.z)
def __sub__(self, other):
return Vector3D(self.x - other.x, self.y - other.y, self.z - other.z)
def dot_product(self, other):
return self.x * other.x + self.y * other.y + self.z * other.z
def cross_product(self, other):
return Vector3D(self.y * other.z - self.z * other.y,
self.z * other.x - self.x * other.z,
self.x * other.y - self.y * other.x)
```
这个类包含了一个构造函数和四个方法:
- `__init__(self, x, y, z)`:构造函数,用于初始化向量的三个分量。
- `__add__(self, other)`:重载加法运算符,用于向量的加法。
- `__sub__(self, other)`:重载减法运算符,用于向量的减法。
- `dot_product(self, other)`:点乘方法,用于计算两个向量的点乘积。
- `cross_product(self, other)`:叉乘方法,用于计算两个向量的叉乘积。
使用示例:
```python
# 创建两个向量
v1 = Vector3D(1, 2, 3)
v2 = Vector3D(4, 5, 6)
# 向量加法
v3 = v1 + v2
print(v3.x, v3.y, v3.z) # 输出:5 7 9
# 向量减法
v4 = v2 - v1
print(v4.x, v4.y, v4.z) # 输出:3 3 3
# 向量点乘
dot_product = v1.dot_product(v2)
print(dot_product) # 输出:32
# 向量叉乘
v5 = v1.cross_product(v2)
print(v5.x, v5.y, v5.z) # 输出:(-3, 6, -3)
```
阅读全文
相关推荐










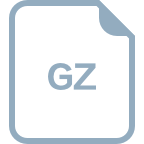

