ffmpeg+sdl 实现音频播放
时间: 2023-10-29 17:07:25 浏览: 54
要使用FFmpeg和SDL实现音频播放,您可以按照以下步骤进行操作:
1. 首先,确保您已经安装了FFmpeg和SDL库。
2. 创建一个C/C++源文件,并包含必要的头文件:
```c
#include <stdio.h>
#include <SDL2/SDL.h>
#include <libavformat/avformat.h>
#include <libavutil/opt.h>
#include <libswresample/swresample.h>
```
3. 初始化FFmpeg和SDL库:
```c
av_register_all();
avformat_network_init();
SDL_Init(SDL_INIT_AUDIO);
```
4. 打开音频文件并解码音频流:
```c
AVFormatContext *formatCtx = NULL;
if (avformat_open_input(&formatCtx, "audio.mp3", NULL, NULL) != 0) {
// 处理打开文件失败的情况
return -1;
}
if (avformat_find_stream_info(formatCtx, NULL) < 0) {
// 处理获取流信息失败的情况
return -1;
}
int audioStreamIndex = -1;
for (int i = 0; i < formatCtx->nb_streams; i++) {
if (formatCtx->streams[i]->codecpar->codec_type == AVMEDIA_TYPE_AUDIO) {
audioStreamIndex = i;
break;
}
}
if (audioStreamIndex == -1) {
// 处理没有找到音频流的情况
return -1;
}
AVCodecParameters *codecPar = formatCtx->streams[audioStreamIndex]->codecpar;
AVCodec *codec = avcodec_find_decoder(codecPar->codec_id);
if (codec == NULL) {
// 处理找不到解码器的情况
return -1;
}
AVCodecContext *codecCtx = avcodec_alloc_context3(codec);
if (avcodec_parameters_to_context(codecCtx, codecPar) < 0) {
// 处理解码器参数设置失败的情况
return -1;
}
if (avcodec_open2(codecCtx, codec, NULL) < 0) {
// 处理打开解码器失败的情况
return -1;
}
```
5. 配置SDL音频参数:
```c
SDL_AudioSpec wantedSpec, obtainedSpec;
wantedSpec.freq = codecCtx->sample_rate;
wantedSpec.format = AUDIO_S16SYS;
wantedSpec.channels = codecCtx->channels;
wantedSpec.silence = 0;
wantedSpec.samples = 1024;wantedSpec.callback = audio_callback;
wantedSpec.userdata = codecCtx;
SDL_OpenAudio(&wantedSpec, &obtainedSpec);
SDL_PauseAudio(0);
```
6. 实现SDL音频回调函数:
```c
void audio_callback(void *userdata, Uint8 *stream, int len) {
AVCodecContext *codecCtx = (AVCodecContext *)userdata;
AVPacket packet;
static uint8_t audio_buffer[(192000 * 3) / 2];
static unsigned int audio_buffer_size = 0;
static unsigned int audio_buffer_index = 0;
while (len > 0) {
if (audio_buffer_index >= audio_buffer_size) {
int ret = av_read_frame(formatCtx, &packet);
if (ret < 0) {
// 处理读取音频帧失败的情况
break;
}
if (packet.stream_index == audioStreamIndex) {
ret = avcodec_send_packet(codecCtx, &packet);
if (ret < 0) {
// 处理发送音频帧给解码器失败的情况
break;
}
ret = avcodec_receive_frame(codecCtx, &frame);
if (ret < 0) {
// 处理接收解码后的音频帧失败的情况
break;
}
int data_size = av_samples_get_buffer_size(NULL, codecCtx->channels,
frame->nb_samples,
codecCtx->sample_fmt, 1);
memcpy(audio_buffer, frame->data[0], data_size);
audio_buffer_size = data_size;
audio_buffer_index = 0;
}
av_packet_unref(&packet);
}
int remaining = audio_buffer_size - audio_buffer_index;
int to_copy = len > remaining ? remaining : len;
memcpy(stream, audio_buffer + audio_buffer_index, to_copy);
len -= to_copy;
stream += to_copy;
audio_buffer_index += to_copy;
}
}
```
7. 清理资源并关闭SDL和FFmpeg库:
```c
SDL_CloseAudio();
SDL_Quit();
avformat_close_input(&formatCtx);
avcodec_free_context(&codecCtx);
return 0;
```
以上是一个简单的示例,演示了如何使用FFmpeg和SDL实现音频播放。您可以根据自己的需求进行调整和扩展。
相关推荐
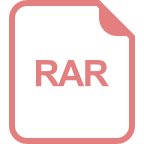
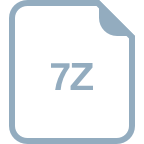














