python最短路径算法dijkstra
时间: 2023-11-21 08:58:45 浏览: 110
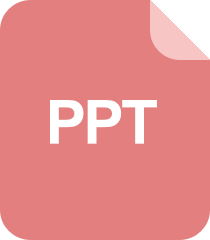
最短路径dijkstra算法
以下是Python实现Dijkstra算法求最短路径的示例代码:
```python
import heapq
def dijkstra(graph, start):
# 初始化距离字典
distances = {vertex: float('infinity') for vertex in graph}
distances[start] = 0
# 初始化堆
heap = [(0, start)]
while heap:
# 弹出堆中最小距离的节点
current_distance, current_vertex = heapq.heappop(heap)
# 如果当前节点已经被处理过,则跳过
if current_distance > distances[current_vertex]:
continue
# 遍历当前节点的邻居节点
for neighbor, weight in graph[current_vertex].items():
distance = current_distance + weight
# 如果新的距离比原来的距离更短,则更新距离字典和堆
if distance < distances[neighbor]:
distances[neighbor] = distance
heapq.heappush(heap, (distance, neighbor))
return distances
# 示例图
graph = {
'A': {'B': 2, 'C': 1},
'B': {'A': 2, 'D': 5},
'C': {'A': 1, 'D': 1, 'E': 3},
'D': {'B': 5, 'C': 1, 'E': 1, 'F': 2},
'E': {'C': 3, 'D': 1, 'F': 1},
'F': {'D': 2, 'E': 1}
}
# 求A到其他节点的最短路径
distances = dijkstra(graph, 'A')
print(distances)
# 输出:{'A': 0, 'B': 2, 'C': 1, 'D': 2, 'E': 3, 'F': 3}
```
阅读全文
相关推荐






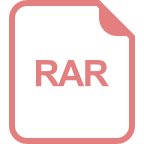
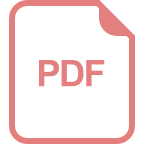
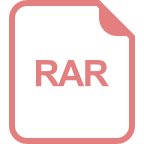
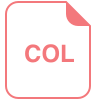
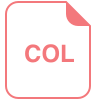
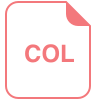



