写一个扫雷小程序,使用python
时间: 2024-06-12 11:06:39 浏览: 104
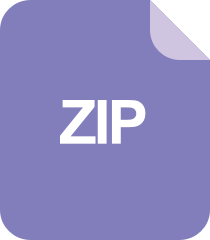
python编写的扫雷小游戏,包括源文件和打包好的可执行exe文件
这是一个简单的扫雷小程序:
```python
import random
def create_board(rows, cols, bombs):
# 创建一个二维列表表示扫雷游戏板
board = [[0 for i in range(cols)] for j in range(rows)]
# 在随机位置放置炸弹
bomb_count = 0
while bomb_count < bombs:
row = random.randint(0, rows-1)
col = random.randint(0, cols-1)
if board[row][col] != -1:
board[row][col] = -1
bomb_count += 1
# 填充数字,表示周围的炸弹数量
for row in range(rows):
for col in range(cols):
if board[row][col] == -1:
continue
count = 0
for i in range(max(0, row-1), min(rows, row+2)):
for j in range(max(0, col-1), min(cols, col+2)):
if board[i][j] == -1:
count += 1
board[row][col] = count
return board
def play_game(board):
rows = len(board)
cols = len(board[0])
uncovered = [[False for i in range(cols)] for j in range(rows)]
game_over = False
while not game_over:
print_board(board, uncovered)
row = int(input("请输入行号:"))
col = int(input("请输入列号:"))
if board[row][col] == -1:
print("你输了!")
game_over = True
else:
uncovered[row][col] = True
if all([all(row) for row in uncovered]):
print("你赢了!")
game_over = True
def print_board(board, uncovered):
rows = len(board)
cols = len(board[0])
print(" ", end="")
for col in range(cols):
print(col, end=" ")
print()
print(" +" + "-" * cols * 2 + "+")
for row in range(rows):
print(row, "|", end="")
for col in range(cols):
if uncovered[row][col]:
if board[row][col] == -1:
print("* ", end="")
else:
print(str(board[row][col]) + " ", end="")
else:
print(". ", end="")
print("|")
print(" +" + "-" * cols * 2 + "+")
rows = 8
cols = 8
bombs = 10
board = create_board(rows, cols, bombs)
play_game(board)
```
该程序使用了两个函数:`create_board` 和 `play_game`。
`create_board` 函数用于创建一个二维列表表示扫雷游戏板。它接受三个参数:行数、列数和炸弹数量。它首先创建一个全是零的二维列表,然后在随机位置放置炸弹。接下来,它遍历整个列表,对于每个位置,计算周围的炸弹数量,并填充到列表中。最后返回该列表。
`play_game` 函数用于玩扫雷游戏。它接受一个参数:扫雷游戏板。它首先创建另一个二维列表,用于跟踪哪些位置已经被揭开。然后,它进入一个循环,直到游戏结束。在每个循环迭代中,它打印出当前游戏板和要求玩家输入行号和列号。如果玩家选择了一个炸弹位置,则游戏结束并打印出“你输了!”。否则,该位置被揭开并在二维列表中标记为已揭开。如果所有位置都已揭开,则游戏结束并打印出“你赢了!”。
`print_board` 函数用于打印当前游戏板。它接受两个参数:游戏板和已揭开的位置。它首先打印列号,然后打印一个分隔线。接下来,它遍历整个游戏板,并根据游戏板和已揭开的位置打印出每个位置的内容。最后,它再次打印一个分隔线。
在主程序中,我们首先定义了行数、列数和炸弹数量。然后,我们使用 `create_board` 函数创建一个扫雷游戏板,并使用 `play_game` 函数玩扫雷游戏。
阅读全文
相关推荐
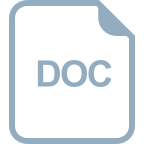
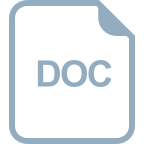

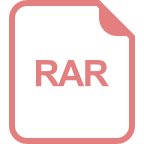









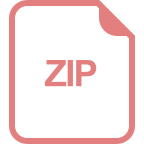
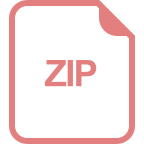

