如何用c++实现求最大网络流
时间: 2024-03-09 16:45:12 浏览: 68
求最大网络流的经典算法是Ford-Fulkerson算法,它的核心思想是不断寻找增广路径,并更新残留网络,直到不存在增广路径为止。以下是使用C++实现Ford-Fulkerson算法求解最大网络流的示例代码:
```cpp
#include <iostream>
#include <queue>
#include <cstring>
using namespace std;
#define MAX_VERTEX_NUM 100 // 最大顶点数
#define INF 0x7fffffff // 无穷大
// 定义邻接矩阵结构体
typedef struct {
int matrix[MAX_VERTEX_NUM][MAX_VERTEX_NUM]; // 邻接矩阵数组
int vertex_num; // 顶点数
int edge_num; // 边数
} AdjMatrix;
// 初始化邻接矩阵
void initAdjMatrix(AdjMatrix* graph, int vertex_num) {
graph->vertex_num = vertex_num;
graph->edge_num = 0;
for (int i = 0; i < vertex_num; i++) {
for (int j = 0; j < vertex_num; j++) {
graph->matrix[i][j] = 0;
}
}
}
// 添加有向边
void addDirectedEdge(AdjMatrix* graph, int from, int to, int weight) {
graph->matrix[from][to] = weight;
graph->edge_num++;
}
// Ford-Fulkerson算法求最大网络流
int maxFlow(AdjMatrix* graph, int source, int sink) {
int n = graph->vertex_num;
// 创建残留网络
int residual[MAX_VERTEX_NUM][MAX_VERTEX_NUM];
memcpy(residual, graph->matrix, sizeof(residual));
int parent[MAX_VERTEX_NUM];
int max_flow = 0;
while (true) {
// 使用BFS寻找增广路径
memset(parent, -1, sizeof(parent));
queue<int> q;
q.push(source);
parent[source] = source;
while (!q.empty()) {
int u = q.front();
q.pop();
for (int v = 0; v < n; v++) {
if (parent[v] == -1 && residual[u][v] > 0) {
parent[v] = u;
q.push(v);
}
}
}
// 如果不存在增广路径,则退出循环
if (parent[sink] == -1) {
break;
}
// 计算增广路径上的最小流量
int path_flow = INF;
for (int v = sink; v != source; v = parent[v]) {
int u = parent[v];
path_flow = min(path_flow, residual[u][v]);
}
// 更新残留网络,并累加最大流量
for (int v = sink; v != source; v = parent[v]) {
int u = parent[v];
residual[u][v] -= path_flow;
residual[v][u] += path_flow;
}
max_flow += path_flow;
}
return max_flow;
}
int main() {
AdjMatrix graph;
initAdjMatrix(&graph, 6);
addDirectedEdge(&graph, 0, 1, 16);
addDirectedEdge(&graph, 0, 2, 13);
addDirectedEdge(&graph, 1, 2, 10);
addDirectedEdge(&graph, 1, 3, 12);
addDirectedEdge(&graph, 2, 1, 4);
addDirectedEdge(&graph, 2, 4, 14);
addDirectedEdge(&graph, 3, 2, 9);
addDirectedEdge(&graph, 3, 5, 20);
addDirectedEdge(&graph, 4, 3, 7);
addDirectedEdge(&graph, 4, 5, 4);
int source = 0;
int sink = 5;
int max_flow = maxFlow(&graph, source, sink);
cout << "最大网络流为:" << max_flow << endl;
return 0;
}
```
以上代码中,使用邻接矩阵存储图的结构,使用Ford-Fulkerson算法求解最大网络流。主函数中构造了一个有向图,然后调用maxFlow函数求解最大网络流,并输出结果。
阅读全文
相关推荐

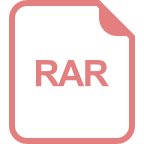







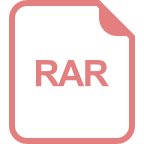
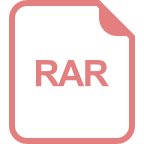

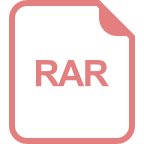




