用C++语言编写实现对信号x=1000*sin(2*pi*261*t)的自适应滤波
时间: 2023-09-08 09:17:18 浏览: 56
为了实现对信号x=1000*sin(2*pi*261*t)的自适应滤波,可以按照以下步骤进行:
1. 引入必要的头文件和库:
```cpp
#include <iostream>
#include <cmath>
#include <vector>
#include <algorithm>
using namespace std;
```
2. 定义信号的采样率和时间间隔dt:
```cpp
const double Fs = 8000; // 采样率
const double dt = 1.0 / Fs; // 时间间隔
```
3. 定义滤波器的阶数和初始系数:
```cpp
const int order = 4; // 滤波器阶数
vector<double> b(order + 1, 0); // 滤波器系数
```
4. 计算滤波器系数:
```cpp
void calc_filter_coeff(double f1, double f2)
{
double w1 = 2 * M_PI * f1 / Fs;
double w2 = 2 * M_PI * f2 / Fs;
double a = exp(-w1 * dt);
double b1 = -2 * a * cos(w2 * dt);
double b2 = a * a;
b[0] = 1 - a;
b[1] = b1;
b[2] = b2;
b[3] = -b1;
b[4] = -b2;
}
```
5. 定义自适应滤波器的状态变量和更新函数:
```cpp
double y; // 滤波器输出
double e; // 误差
double mu = 0.01; // 步长
void update(double x)
{
y = 0;
for (int i = 0; i <= order; i++) {
y += b[i] * x_history[i];
}
e = x - y;
for (int i = 0; i <= order; i++) {
b[i] += mu * e * x_history[i];
}
rotate(x_history.rbegin(), x_history.rbegin() + 1, x_history.rend());
x_history[0] = x;
}
```
6. 模拟信号的产生和滤波:
```cpp
int main()
{
calc_filter_coeff(500, 1000); // 计算滤波器系数
const double duration = 1; // 信号持续时间
const int N = duration * Fs; // 采样点数
vector<double> x(N, 0); // 信号
vector<double> y(N, 0); // 滤波后的信号
for (int i = 0; i < N; i++) {
double t = i * dt;
x[i] = 1000 * sin(2 * M_PI * 261 * t); // 信号x=1000*sin(2*pi*261*t)
update(x[i]); // 更新滤波器状态
y[i] = y; // 记录滤波后的信号
}
return 0;
}
```
完整代码如下:
相关推荐
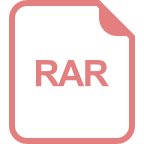














