用python语言,用AES算法实现对文件的加密与解密过程
时间: 2024-10-09 16:01:49 浏览: 33
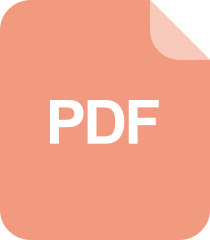
Python基于pycrypto实现的AES加密和解密算法示例
在Python中,我们可以使用`cryptography`库来实现AES(Advanced Encryption Standard)算法的文件加密和解密。以下是简单的步骤:
**安装所需库**:
```bash
pip install cryptography
```
**导入必要的模块**:
```python
from Crypto.Cipher import AES
from Crypto.Util.Padding import pad, unpad
import os
```
**设置秘钥和初始向量(IV)**:
```python
key = b'sixteen_byte_key' # 选择一个16字节的秘钥
iv = b'twelve_byte_iv' # 选择一个16字节的初始向量,通常从随机生成
```
**加密函数**:
```python
def encrypt_file(file_path, key, iv):
with open(file_path, 'rb') as f_in:
data = f_in.read()
cipher = AES.new(key, AES.MODE_CBC, iv)
encrypted_data = cipher.encrypt(pad(data, AES.block_size))
return cipher.iv + encrypted_data
```
**解密函数**:
```python
def decrypt_file(encrypted_file, key, iv):
with open(encrypted_file, 'rb') as f_in:
encrypted_data = f_in.read()
iv = encrypted_data[:16] # 提取IV
encrypted_data = encrypted_data[16:] # 去除IV
cipher = AES.new(key, AES.MODE_CBC, iv)
decrypted_data = unpad(cipher.decrypt(encrypted_data), AES.block_size)
return decrypted_data
```
**示例**:
```python
# 加密
encrypted_data = encrypt_file('input.txt', key, iv)
# 写入到新的加密文件
with open('output.enc', 'wb') as f_out:
f_out.write(encrypted_data)
# 解密
original_data = decrypt_file('output.enc', key, iv)
with open('decrypted.txt', 'wb') as f_out:
f_out.write(original_data)
```
阅读全文
相关推荐
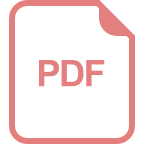
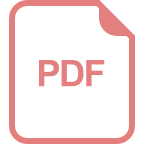















