#include <iostream> #include <string.h> #include <cstdio> #include <queue> #define N 105 using namespace std; const int xx[6] = {-1, 1, 0, 0, 0, 0}; const int yy[6] = {0, 0, -1, 1, 0, 0}; const int zz[6] = {0, 0, 0, 0, -1, 1}; int map[N][N][N], l, r, c, bx, by, bz, ex, ey, ez, ans; char ch; struct node { int x, y, z; }; queue <node> q; int main() { while(cin >> l >> r >> c && l && r && c) { memset(map, 0, sizeof(map)); ans = 0; for(int i = 1; i <= l; ++i) for(int j = 1; j <= r; ++j) for(int k = 1; k <= c4; ++k) { cin >> ch; if(ch == '\n') --k; else if(ch == 'S') { bx = j; by = k; bz = i; } else if(ch == 'E') { ex = j; ey = k; ez = i; } else if(ch == '#') map[i][j][k] = 1; } node bxyz; bxyz.x = bx; bxyz.y = by; bxyz.z = bz; map[bz][bx][by] = 1; q.push(bxyz); bool f = 1; while(!q.empty()) { int len = q.size(); ++ans; for(int i = 1; i <= len; ++i) { int x = q.front().x; int y = q.front().y; int z = q.front().z; q.pop(); for(int j = 0; j < 6; ++j) { int nx = x + xx[j]; int ny = y + yy[j]; int nz = z + zz[j]; if(nx > 0 && nx <= r && ny > 0 && ny <= c && nz > 0 && nz <= l && !map[nz][nx][ny]) { map[nz][nx][ny] = 1; node nxyz; nxyz.x = nx; nxyz.y = ny; nxyz.z = nz; q.push(nxyz); if(nx == ex && ny == ey && nz == ez) { printf("Escaped in %d minute(s).\n", ans); f = 0; break; } } } if(!f) break; } if(!f) break; } if(f) printf("Trapped!\n"); } return 0; }
时间: 2024-04-09 11:30:52 浏览: 157
这段代码是一个三维迷宫问题的求解程序。使用了广度优先搜索算来找到从起点到终点的最短路径。
代码中的map`数组表示迷宫地图,其中1表示墙壁,0可通行的路径。点和终点分别用'S'和'E'表示。
程序首先读取迷宫的长、宽和高。然后根据输入构建迷宫地图。接下来,将起点加入队列,并将其标记为已访问。然后开始进行广度优先搜索,每次从队列中取出一个节点,并将其周围未访问过的相邻节点加入队列。如果遇到终点节点,输出最短路径的长度,并结束搜索。如果队列为空仍未找到终点节点,则输出"Trapped!"。
该程序可以多次运行,每次输入一个迷宫地图。当输入的长、宽和高都为0时,程序结束。
需要注意的是,这段代码存在一些语法错误,如第30行的"c4"应为"c"。另外,该程序使用了C++标准库的输入输出流操作,因此需要包含相关头文件。修复这些错误后,代码应该可以正确运行。
相关问题
#include<cstdio> #include<cstdlib> #include<cstring> #include<cmath> #include<ctime> #include<iostream> #include<algorithm> #include<map> #include<stack> #include<queue> #include<vector> #include<set> #include<string> #define dd double #define ll long long dd PI = acos(-1); using namespace std; const ll MAXN = 1e5 + 5; const ll INF = 1e9 + 5; ll n; struct node { ll x, y; }s[5000]; ll dp[MAXN] = { 0 }; int main() { //ios::sync_with_stdio(false); ll n, m; cin >> n >> m; for (ll i = 1; i <= n; i++) { cin >> s[i].x >> s[i].y; } for (ll i = 1; i <= n; i++) { for (ll j = m; j >= s[i].x; j--) { dp[j] = max(dp[j], dp[j - s[i].x] + s[i].y); } } cout << dp[m] << endl; }
这是一段动态规划求解背包问题的代码,用来求解一个容量为m的背包能够装下的最大价值。具体实现过程为:
1. 定义一个结构体node,用来存储物品的重量和价值。
2. 读入物品数量n和背包容量m,然后读入每个物品的重量和价值。
3. 使用二重循环,遍历每一个物品,然后从大到小枚举背包容量j,更新dp[j]的值,表示容量为j的背包能够装下的最大价值。
4. 最后输出dp[m]的值,即容量为m的背包能够装下的最大价值。
值得注意的是,这段代码中还定义了一些常量和宏定义,如MAXN、INF等,以及一些头文件和命名空间的使用。
#include <cstdio> #include<iostream> #include<queue> #include<algorithm> #include<cstring> #include<iomanip> #define inf 0x3f3f3f3f3f3f3f3fLL using namespace std; typedef long long ll; const int N=1e6; int n,k; int a[N]; int quick(int l,int r,int k) { if(l==r) return a[l]; int i=l-1,j=r+1,x=a[l]; while(i<j) { while(a[i++]<x); while(a[j--]>x); if(i<j) swap(a[i],a[j]); } int s=j-l+1; if(k<=s) return quick(l,j,k); return quick(j+1,r,k-s); } int main() { cin>>n>>k; for(int i=0;i<n;i++) { cin>>a[i]; } cout<<quick(0,n-1,k); return 0; }上述代码为什么报错
代码中存在一些问题,可能导致编译器报错。具体问题可见以下解释:
1. 缺少头文件
代码开头只有一行 `#include <cstdio>`,缺少其他可能需要的头文件,如 `iostream`、`queue`、`algorithm`、`cstring` 等。
可以添加以下代码:
```c++
#include<iostream>
#include<queue>
#include<algorithm>
#include<cstring>
#include<iomanip>
```
2. 数组大小
定义了数组 `a` 后,没有指定其大小,应该将数组大小指定为常量 `N`,即 `int a[N]`。
3. 变量未定义
在主函数中使用了 `n` 和 `k` 两个变量,但是并没有给它们赋初值,需要在输入时进行初始化。
4. 函数返回类型错误
定义了 `quick` 函数,但是没有给出返回类型,应该指定其返回类型为 `int`。
5. 函数参数类型错误
定义了 `quick` 函数,但是其参数类型应该为 `int l, int r, int k`,而不是 `int left, int right, int k`。
6. 函数内部变量未定义
在 `quick` 函数中,定义了变量 `i` 和 `j`,但是没有给出初始值,这可能导致编译器报错。可以将其初始化为 `l-1` 和 `r+1`。
7. 函数返回值错误
在 `quick` 函数中,当 `k<=s` 时应该返回 `quick(l,j,k)`,而不是 `quick(left,j,k)`。同理,当 `k>s` 时应该返回 `quick(j+1,r,k-s)`,而不是 `quick(j+1,right,k-s)`。
修改完上述问题后的代码如下:
```c++
#include <iostream>
#include <queue>
#include <algorithm>
#include <cstring>
#include <iomanip>
#define inf 0x3f3f3f3f3f3f3f3fLL
#define N 1000000
using namespace std;
typedef long long ll;
int n,k;
int a[N];
int quick(int l,int r,int k) {
if(l==r) return a[l];
int i=l-1,j=r+1,x=a[l];
while(i<j) {
while(a[++i]<x);
while(a[--j]>x);
if(i<j) swap(a[i],a[j]);
}
int s=j-l+1;
if(k<=s) return quick(l,j,k);
return quick(j+1,r,k-s);
}
int main() {
cin>>n>>k;
for(int i=0;i<n;i++) {
cin>>a[i];
}
cout<<quick(0,n-1,k);
return 0;
}
```
阅读全文
相关推荐
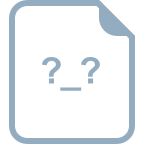
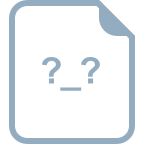


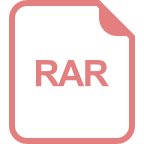
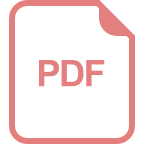



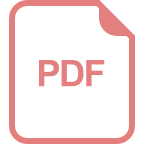
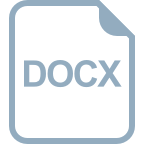
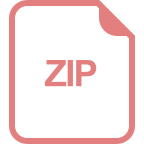
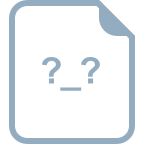
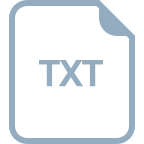