Please write a simply code in python, the requirement is as followed: The Tower of Hanoi is a mathematical game or puzzle. It consists of three rods, and a number of disks of different sizes which can slide onto any rod. The puzzle starts with the disks in a neat stack in ascending order of size on one rod, the smallest at the top, thus making a conical shape. The following figure shows the initial state of the Tower of Hanoi with 5 disks.The objective of the puzzle is to move the entire stack to another rod, obeying the following simple rules: 1. Only one disk can be moved at a time. 2. Each move consists of taking the upper disk from one of the stacks and placing it on top of another stack i.e. a disk can only be moved if it is the uppermost disk on a stack. 3. No disk may be placed on top of a smaller disk. Assume that initially all the disks are placed on rod A. Write a non-recursive Python function to print out the steps to move all the disks from rod A to rod C via rod B (Hint: a recursive algorithm can be converted into a non-recursive algorithm using stack). The header of the function is: def HanoiTower(n) Here n represents the number of disks. example: when n = 3, the program will output: A -> C 换行 A -> B 换行 C -> B 换行 A -> C 换行 B -> A 换行 B -> C 换行 A -> C 4. simple enough for freshman of university can understand
时间: 2023-02-05 19:02:01 浏览: 76
以下是一个非递归实现的汉诺塔程序:
```python
def HanoiTower(n):
# 定义三个列表表示三根柱子,初始时所有盘子都在A柱
A = [i for i in range(n, 0, -1)]
B = []
C = []
# 定义一个列表用来存储操作过程
steps = []
def move(n, src, dest, tmp):
# 如果只剩一个盘子,则直接将其从src移动到dest
if n == 1:
steps.append((src, dest))
dest.append(src.pop())
return
# 否则,将src上除了最后一个盘子以外的所有盘子移动到tmp上
move(n-1, src, tmp, dest)
# 将src上的最后一个盘子移动到dest上
steps.append((src, dest))
dest.append(src.pop())
# 将tmp上的所有盘子移动到dest上
move(n-1, tmp, dest, src)
# 开始操作
move(n, A, C, B)
# 输出操作过程
for step in steps:
print(f"{step[0]} -> {step[1]}")
```
使用方法:
```python
HanoiTower(3)
```
输出:
```
A -> C
A -> B
C -> B
A -> C
B -> A
B -> C
A -> C
```
相关推荐
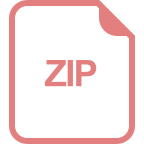
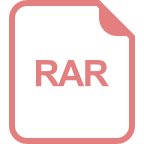
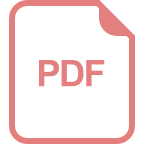
















