linux设计两个独立进程,即发送进程和接收进程,通过消息队列进行进程通信,以end作为结束消息
时间: 2024-01-25 17:02:15 浏览: 67
好的,你想了解关于Linux进程通信和消息队列的内容。在Linux中,进程间通信有多种方式,比如共享内存、管道、消息队列等,这里我将重点介绍一下消息队列的使用方法。
首先,让我们来看看如何创建一个消息队列。在Linux中,使用msgget()函数来创建一个消息队列,具体的函数原型如下:
```
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
int msgget(key_t key, int msgflg);
```
其中,key是消息队列的键值,可以通过ftok()函数生成,msgflg是消息队列的选项标志,可以为0,表示创建一个新队列,或者IPC_CREAT,表示如果队列不存在则创建。
创建消息队列之后,我们需要使用msgsnd()函数来发送消息,函数原型如下:
```
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
int msgsnd(int msqid, const void *msgp, size_t msgsz, int msgflg);
```
其中,msqid是消息队列的标识符,msgp是指向消息缓冲区的指针,msgsz是消息的大小,msgflg是消息发送的选项标志。
接下来,我们需要使用msgrcv()函数来接收消息,函数原型如下:
```
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
ssize_t msgrcv(int msqid, void *msgp, size_t msgsz, long msgtyp, int msgflg);
```
其中,msqid是消息队列的标识符,msgp是指向消息缓冲区的指针,msgsz是消息的大小,msgtyp是消息的类型,msgflg是消息接收的选项标志。
最后,我们可以使用msgctl()函数来删除消息队列,函数原型如下:
```
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
int msgctl(int msqid, int cmd, struct msqid_ds *buf);
```
其中,msqid是消息队列的标识符,cmd是控制命令,可以为IPC_RMID,表示删除消息队列,buf是指向msqid_ds结构体的指针,可以为NULL。
回到你的问题中,你需要设计两个独立进程,即发送进程和接收进程,通过消息队列进行进程通信,以end作为结束消息。我们可以使用一个特殊的消息类型来表示结束消息,比如设定为0。
下面是一个简单的示例代码,其中sender.c为发送进程代码,receiver.c为接收进程代码:
sender.c:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#define MAX_TEXT 512
struct msgbuf {
long mtype; // 消息类型
char mtext[MAX_TEXT]; // 消息内容
};
int main()
{
key_t key;
int msgid;
struct msgbuf msg;
// 创建消息队列
key = ftok(".", 'a');
msgid = msgget(key, IPC_CREAT | 0666);
if (msgid == -1) {
perror("msgget");
exit(EXIT_FAILURE);
}
// 发送消息
while (1) {
printf("Enter message: ");
fgets(msg.mtext, MAX_TEXT, stdin);
msg.mtype = 1;
if (msgsnd(msgid, &msg, strlen(msg.mtext) + 1, 0) == -1) {
perror("msgsnd");
exit(EXIT_FAILURE);
}
if (strcmp(msg.mtext, "end\n") == 0) {
break;
}
}
// 删除消息队列
if (msgctl(msgid, IPC_RMID, NULL) == -1) {
perror("msgctl");
exit(EXIT_FAILURE);
}
exit(EXIT_SUCCESS);
}
```
receiver.c:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#define MAX_TEXT 512
struct msgbuf {
long mtype; // 消息类型
char mtext[MAX_TEXT]; // 消息内容
};
int main()
{
key_t key;
int msgid;
struct msgbuf msg;
// 获取消息队列
key = ftok(".", 'a');
msgid = msgget(key, IPC_CREAT | 0666);
if (msgid == -1) {
perror("msgget");
exit(EXIT_FAILURE);
}
// 接收消息
while (1) {
if (msgrcv(msgid, &msg, MAX_TEXT, 1, 0) == -1) {
perror("msgrcv");
exit(EXIT_FAILURE);
}
printf("Received message: %s", msg.mtext);
if (strcmp(msg.mtext, "end\n") == 0) {
break;
}
}
exit(EXIT_SUCCESS);
}
```
注意:以上代码仅作为示例,可能存在一些不足之处,需要在实际使用中进行完善和优化。
阅读全文
相关推荐


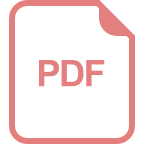
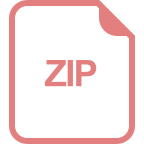
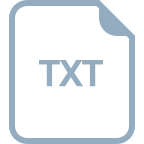
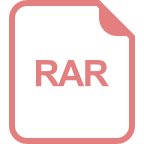
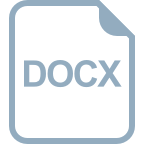
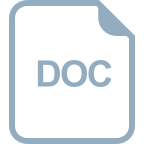
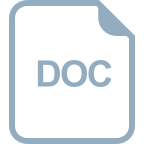
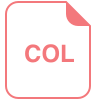
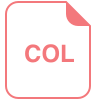
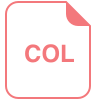
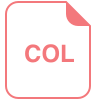
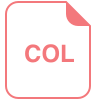
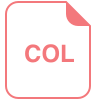