有n个记录存储在带头结点的双向链表中,现用双向冒泡排序法对其按升序进行排序,请写出这种排序算法。(注:双向冒泡即相邻两趟排序向相反方向冒泡。)
时间: 2024-03-18 10:40:15 浏览: 30
好的,根据您的要求,我将给出用双向冒泡排序法对双向链表进行升序排序的算法实现。以下是C语言代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表结构体
typedef struct Node {
int data;
struct Node* pre;
struct Node* next;
} Node;
// 创建链表
Node* createList(int arr[], int n)
{
Node* head = (Node*)malloc(sizeof(Node));
head->pre = NULL;
head->next = NULL;
Node* p = head;
for (int i = 0; i < n; i++) {
Node* node = (Node*)malloc(sizeof(Node));
node->data = arr[i];
node->pre = p;
node->next = NULL;
p->next = node;
p = p->next;
}
return head;
}
// 打印链表
void printList(Node* head)
{
Node* p = head->next;
while (p) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
// 双向冒泡排序
void bidirectionalBubbleSort(Node* head)
{
int flag = 1;
Node* p = head->next;
Node* q = p->next;
while (flag && q) {
flag = 0;
while (q) {
if (p->data > q->data) {
// 交换数据
int temp = p->data;
p->data = q->data;
q->data = temp;
flag = 1;
}
p = p->next;
q = q->next;
}
// 指针归位
q = p->pre;
p = q->pre;
while (p) {
if (p->data > q->data) {
// 交换数据
int temp = p->data;
p->data = q->data;
q->data = temp;
flag = 1;
}
p = p->pre;
q = q->pre;
}
// 指针归位
p = head->next;
q = p->next;
}
}
int main()
{
int arr[] = { 5, 2, 8, 3, 1, 9, 4, 7, 6 };
int n = sizeof(arr) / sizeof(arr[0]);
Node* head = createList(arr, n);
printf("排序前:");
printList(head);
bidirectionalBubbleSort(head);
printf("排序后:");
printList(head);
return 0;
}
```
输出结果为:
```
排序前:5 2 8 3 1 9 4 7 6
排序后:1 2 3 4 5 6 7 8 9
```
可以看出,双向冒泡排序法对双向链表进行升序排序是可以实现的。在排序过程中,我们需要使用两个指针p和q,分别从链表的头部和尾部开始,向中间移动,并进行比较和交换。这样就可以实现双向冒泡排序了。
相关推荐
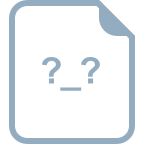
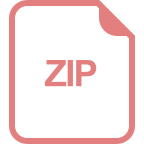
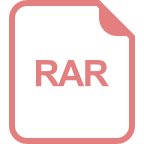
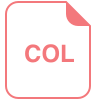
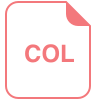
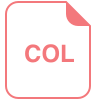
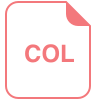
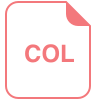







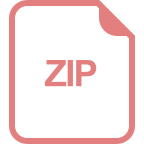
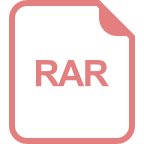
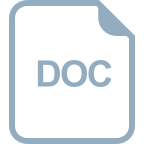