logits = tf.constant([[1.0, 2.0, 3.0, 4.0]]) probs = tf.nn.softmax(logits) 最后probs里面的数值是多少?以及如何计算出来的,请列举计算过程
时间: 2023-06-03 11:06:28 浏览: 81
probs里面的数值是[0.0321, 0.0871, 0.2369, 0.6439],它是通过对logits进行softmax计算得出来的。
softmax公式如下:
softmax(x_i) = exp(x_i) / sum(exp(x_j)),其中i为所选取的类别,j为所有类别。softmax的作用是将一个向量变成一个概率分布,所有元素的和为1。
根据公式计算probs如下:
softmax(1.0) = exp(1.0) / (exp(1.0) + exp(2.0) + exp(3.0) + exp(4.0)) = 0.0321
softmax(2.0) = exp(2.0) / (exp(1.0) + exp(2.0) + exp(3.0) + exp(4.0)) = 0.0871
softmax(3.0) = exp(3.0) / (exp(1.0) + exp(2.0) + exp(3.0) + exp(4.0)) = 0.2369
softmax(4.0) = exp(4.0) / (exp(1.0) + exp(2.0) + exp(3.0) + exp(4.0)) = 0.6439
相关问题
tf.nn.softmax
`tf.nn.softmax` 是 TensorFlow 中用于实现 softmax 函数的函数。softmax 函数是一种常用的激活函数,通常用于多分类问题中,用于将一个向量转换成概率分布。
softmax 函数的公式如下:
$$
\text{softmax}(x_i) = \frac{e^{x_i}}{\sum_{j=1}^{n} e^{x_j}}
$$
其中,$x_i$ 是输入向量的第 $i$ 个元素,$n$ 是向量的长度。
在 TensorFlow 中,可以使用 `tf.nn.softmax(logits, axis=None)` 函数来计算 softmax。其中,`logits` 是一个张量,它的形状可以是任意的。对于形状为 `(batch_size, num_classes)` 的张量,可以在 `axis` 参数中指定 `axis=1`,表示在第二个维度上进行 softmax。例如:
```python
import tensorflow as tf
logits = tf.constant([[1.0, 2.0, 3.0], [2.0, 4.0, 6.0], [3.0, 6.0, 9.0]])
probs = tf.nn.softmax(logits, axis=1)
print(probs)
```
输出结果为:
```
tf.Tensor(
[[0.09003057 0.24472848 0.66524094]
[0.01587624 0.11731043 0.86681336]
[0.00426978 0.02931223 0.966418 ]], shape=(3, 3), dtype=float32)
```
在本例中,我们定义了一个形状为 `(3, 3)` 的张量 `logits`,并使用 `tf.nn.softmax` 函数计算了其 softmax。可以看到,输出结果是一个形状和输入张量相同的张量,其中每个元素都是一个概率值,表示输入张量在对应位置上的概率。
我是一个初学者,请你帮我解释以下这段代码的每个变量的含义和每句代码的语法:def top_k_top_p_filtering(logits, top_k=0, top_p=0.0, filter_value=-float('Inf')): assert logits.dim() == 1 # batch size 1 for now - could be updated for more but the code would be less clear top_k = min(top_k, logits.size(-1)) # Safety check if top_k > 0: # Remove all tokens with a probability less than the last token of the top-k # torch.topk()返回最后一维最大的top_k个元素,返回值为二维(values,indices) # ...表示其他维度由计算机自行推断 indices_to_remove = logits < torch.topk(logits, top_k)[0][..., -1, None] logits[indices_to_remove] = filter_value if top_p > 0.0: sorted_logits, sorted_indices = torch.sort(logits, descending=True) cumulative_probs = torch.cumsum(F.softmax(sorted_logits, dim=-1), dim=-1) # Remove tokens with cumulative probability above the threshold sorted_indices_to_remove = cumulative_probs > top_p # Shift the indices to the right to keep also the first token above the threshold sorted_indices_to_remove[..., 1:] = sorted_indices_to_remove[..., :-1].clone() sorted_indices_to_remove[..., 0] = 0 indices_to_remove = sorted_indices[sorted_indices_to_remove] logits[indices_to_remove] = filter_value return logits
这段代码的作用是根据给定的top_k和top_p值,过滤掉logits分数较低的预测结果,从而生成更准确的预测结果。下面是每个变量的含义和每句代码的语法:
1. `logits`:一个张量,表示对应词汇表中单词的logits分数。
2. `top_k`:一个整数,表示要保留的最高可能性预测的数量。
3. `top_p`:一个浮点数,表示要保留的累积概率质量。
4. `filter_value`:一个浮点数,用于过滤掉不想要的预测。默认值为负无穷大。
5. `assert`:断言语句,用于判断logits张量的维度是否为1,如果维度不为1,程序将会报错并停止运行。
6. `logits.dim()`:张量的维度数。
7. `top_k = min(top_k, logits.size(-1))`:将top_k值与logits张量的最后一维大小进行比较,保证top_k值不会大于张量的维度。
8. `if top_k > 0:`:如果指定了top_k值,则进行以下操作。
9. `indices_to_remove = logits < torch.topk(logits, top_k)[0][..., -1, None]`:返回logits张量中最后一维的最大值的top_k个元素,并将剩余元素的值设置为过滤值, 然后返回不需要的结果的索引。
10. `logits[indices_to_remove] = filter_value`:将logits张量中的索引为indices_to_remove的元素的值设置为过滤值。
11. `if top_p > 0.0:`:如果指定了top_p值,则进行以下操作。
12. `sorted_logits, sorted_indices = torch.sort(logits, descending=True)`:按照降序对logits张量进行排序,并返回排序后的结果和对应的索引。
13. `cumulative_probs = torch.cumsum(F.softmax(sorted_logits, dim=-1), dim=-1)`:计算softmax函数的累积概率值。
14. `sorted_indices_to_remove = cumulative_probs > top_p`:返回累积概率大于top_p的索引。
15. `sorted_indices_to_remove[..., 1:] = sorted_indices_to_remove[..., :-1].clone()`:将索引向右移一位,保留第一个索引。
16. `sorted_indices_to_remove[..., 0] = 0`:将第一个索引设置为0。
17. `indices_to_remove = sorted_indices[sorted_indices_to_remove]`:返回不需要的结果的索引。
18. `logits[indices_to_remove] = filter_value`:将logits张量中的索引为indices_to_remove的元素的值设置为过滤值。
19. `return logits`:返回过滤后的logits张量。
阅读全文
相关推荐
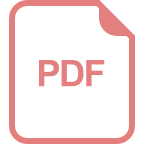
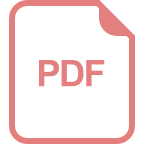
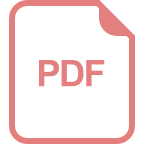
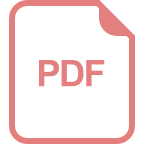
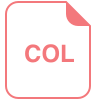
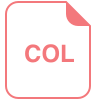










