用c语言写一段代码,功能是增加窗口,窗口数量无限,窗口应有编号、业务类型、排队队列,业务类型包括个人现金,个人其他,对公现金,对公其他。需要标注注释
时间: 2024-01-12 21:02:52 浏览: 26
好的,以下是我给出的一个示例代码,它使用 C 语言实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_WINDOW_NUM 1000 // 最大窗口数
#define MAX_QUEUE_LEN 1000 // 最大队列长度
// 顾客信息
typedef struct {
int customer_id; // 顾客编号
char service_type[20]; // 服务类型
} Customer;
// 窗口信息
typedef struct {
int window_id; // 窗口编号
char service_type[20]; // 服务类型
Customer queue[MAX_QUEUE_LEN]; // 窗口排队队列
int queue_front; // 队首指针
int queue_rear; // 队尾指针
} Window;
// 银行信息
typedef struct {
Window windows[MAX_WINDOW_NUM]; // 窗口列表
int window_num; // 窗口数量
int customer_id_counter; // 顾客编号计数器
} Bank;
// 添加一个窗口
void add_window(Bank *bank, int window_id, const char *service_type) {
if (bank->window_num >= MAX_WINDOW_NUM) {
printf("Error: too many windows\n");
return;
}
Window *window = &bank->windows[bank->window_num];
window->window_id = window_id;
strcpy(window->service_type, service_type);
window->queue_front = window->queue_rear = 0;
bank->window_num++;
printf("Window %d added, service type: %s\n", window_id, service_type);
}
// 添加一个顾客
void add_customer(Window *window, Customer customer) {
if (window->queue_rear >= MAX_QUEUE_LEN) {
printf("Error: queue is full\n");
return;
}
window->queue[window->queue_rear] = customer;
window->queue_rear++;
printf("Customer %d added to window %d, service type: %s\n", customer.customer_id, window->window_id, customer.service_type);
}
// 生成一个新的顾客
Customer next_customer(Bank *bank) {
Customer customer = {++bank->customer_id_counter, ""};
return customer;
}
// 以下是一个示例程序,用于演示如何使用上述函数来创建窗口和添加顾客
int main() {
Bank bank = {{}, 0, 0};
add_window(&bank, 1, "个人现金");
add_window(&bank, 2, "对公现金");
Customer customer1 = next_customer(&bank);
add_customer(&bank.windows[0], customer1);
Customer customer2 = next_customer(&bank);
strcpy(customer2.service_type, "对公其他");
add_customer(&bank.windows[1], customer2);
return 0;
}
```
上述代码中,我们定义了三个结构体:`Customer`、`Window` 和 `Bank`。其中,`Customer` 结构体表示银行的顾客信息,`Window` 结构体表示银行的窗口信息,`Bank` 结构体表示整个银行的信息。
在 `Window` 结构体中,我们使用了一个数组来表示排队队列。`queue_front` 和 `queue_rear` 分别表示队首和队尾指针,可以用来判断队列是否为空或已满。
在 `Bank` 结构体中,我们使用了一个 `window_num` 变量来表示当前窗口数量,`customer_id_counter` 变量用于生成新的顾客编号。
在上述示例程序中,我们首先创建了一个 `Bank` 对象,然后调用了 `add_window` 函数添加了两个窗口,分别提供个人现金和对公现金的服务。接着,我们调用了 `next_customer` 函数生成了两个新的顾客对象,一个加入到了窗口 1 的排队队列中,另一个加入到了窗口 2 的排队队列中。
相关推荐
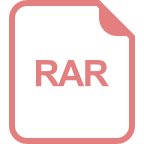

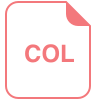
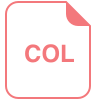
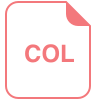
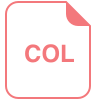









