用pytorch写一个带注意力机制的CNN卷积网络的代码
时间: 2023-06-02 20:06:49 浏览: 59
好的,以下是一个简单的带注意力机制的卷积神经网络的 PyTorch 代码示例:
```
import torch
import torch.nn as nn
class Attention(nn.Module):
def __init__(self, in_channels, out_channels):
super(Attention, self).__init__()
self.query_conv = nn.Conv2d(in_channels, out_channels, kernel_size=1)
self.key_conv = nn.Conv2d(in_channels, out_channels, kernel_size=1)
self.value_conv = nn.Conv2d(in_channels, out_channels, kernel_size=1)
def forward(self, x):
# x shape: (batch_size, in_channels, h, w)
# project input tensor to query, key, and value tensor
query = self.query_conv(x) # (batch_size, out_channels, h, w)
key = self.key_conv(x) # (batch_size, out_channels, h, w)
value = self.value_conv(x) # (batch_size, out_channels, h, w)
# calculate attention weights
key = key.permute(0, 2, 3, 1).contiguous() # (batch_size, h, w, out_channels)
query = query.permute(0, 2, 3, 1).contiguous() # (batch_size, h, w, out_channels)
attn_weights = torch.matmul(query, key.transpose(2, 3))
attn_weights = nn.functional.softmax(attn_weights, dim=-1)
# apply attention weights to value tensor
value = value.permute(0, 2, 3, 1).contiguous() # (batch_size, h, w, out_channels)
attn_values = torch.matmul(attn_weights, value)
attn_values = attn_values.permute(0, 3, 1, 2).contiguous() # (batch_size, out_channels, h, w)
return attn_values
class ConvNet(nn.Module):
def __init__(self):
super(ConvNet, self).__init__()
self.conv1 = nn.Conv2d(3, 32, 3, padding=1)
self.conv2 = nn.Conv2d(32, 64, 3, padding=1)
self.conv3 = nn.Conv2d(64, 128, 3, padding=1)
self.attention1 = Attention(32, 32)
self.attention2 = Attention(64, 64)
self.attention3 = Attention(128, 128)
self.fc = nn.Linear(128 * 4 * 4, 10)
def forward(self, x):
x = nn.functional.relu(self.conv1(x))
x = self.attention1(x) + x
x = nn.functional.relu(nn.functional.max_pool2d(self.conv2(x), 2))
x = self.attention2(x) + x
x = nn.functional.relu(nn.functional.max_pool2d(self.conv3(x), 2))
x = self.attention3(x) + x
x = x.view(-1, 128 * 4 * 4)
x = self.fc(x)
return x
```
这个网络包含了三个卷积层和三个注意力机制,注意力机制被用于加强中间层的特征,以提高模型的准确性。 要注意的是,在实际使用中,这个简单的示例可能需要细微的调整来适应特定的问题。
相关推荐
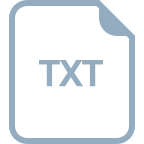
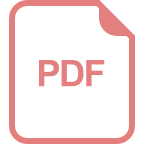














