详细解释一下代码各行的意思import numpy import matplotlib.pyplot as plt from tslearn.clustering import KShape from tslearn.datasets import CachedDatasets from tslearn.preprocessing import TimeSeriesScalerMeanVariance seed = 0 numpy.random.seed(seed) X_train, y_train, X_test, y_test = CachedDatasets().load_dataset("Trace") # Keep first 3 classes and 50 first time series X_train = X_train[y_train < 4] X_train = X_train[:50] numpy.random.shuffle(X_train) # For this method to operate properly, prior scaling is required X_train = TimeSeriesScalerMeanVariance().fit_transform(X_train) sz = X_train.shape[1] # kShape clustering ks = KShape(n_clusters=3, verbose=True, random_state=seed) y_pred = ks.fit_predict(X_train) plt.figure() for yi in range(3): plt.subplot(3, 1, 1 + yi) for xx in X_train[y_pred == yi]: plt.plot(xx.ravel(), "k-", alpha=.2) plt.plot(ks.cluster_centers_[yi].ravel(), "r-") plt.xlim(0, sz) plt.ylim(-4, 4) plt.title("Cluster %d" % (yi + 1)) plt.tight_layout() plt.show()
时间: 2024-04-06 16:29:29 浏览: 58
这段代码是一个时间序列聚类算法 kShape 的实现,以下是每行代码的解释:
1. `import numpy import matplotlib.pyplot as plt from tslearn.clustering import KShape from tslearn.datasets import CachedDatasets from tslearn.preprocessing import TimeSeriesScalerMeanVariance`:导入所需要的模块
2. `seed = 0 numpy.random.seed(seed)`:设置随机种子,以确保结果可重复
3. `X_train, y_train, X_test, y_test = CachedDatasets().load_dataset("Trace")`:使用 CachedDatasets 类载入数据集,数据集名称为 "Trace",返回训练集和测试集的时间序列和对应的标签
4. `X_train = X_train[y_train < 4] X_train = X_train[:50] numpy.random.shuffle(X_train)`:选择前 3 类标签的时间序列,并随机选择前 50 个时间序列进行训练
5. `X_train = TimeSeriesScalerMeanVariance().fit_transform(X_train)`:对训练集进行均值方差缩放
6. `sz = X_train.shape[1]`:获取训练集中时间序列的长度
7. `ks = KShape(n_clusters=3, verbose=True, random_state=seed)`:初始化 kShape 聚类算法模型,设置聚类数目为 3,verbose 参数设置为 True,random_state 参数设置为前面设置的随机种子
8. `y_pred = ks.fit_predict(X_train)`:使用训练集进行模型训练并预测训练集中每个时间序列所属的聚类簇
9. `plt.figure() for yi in range(3): plt.subplot(3, 1, 1 + yi) for xx in X_train[y_pred == yi]: plt.plot(xx.ravel(), "k-", alpha=.2) plt.plot(ks.cluster_centers_[yi].ravel(), "r-") plt.xlim(0, sz) plt.ylim(-4, 4) plt.title("Cluster %d" % (yi + 1)) plt.tight_layout() plt.show()`:画出聚类结果的可视化图像,其中,for 循环遍历每个聚类簇,xx 为该聚类簇中的时间序列,通过 plt.plot() 函数画出该时间序列,ks.cluster_centers_ 为聚类簇的中心点,也通过 plt.plot() 函数画出。plt.xlim() 和 plt.ylim() 分别设置 x 轴和 y 轴的范围。plt.title() 为子图设置标题。最后,使用 plt.tight_layout() 函数调整子图的大小和位置,并使用 plt.show() 函数显示图像。
阅读全文
相关推荐
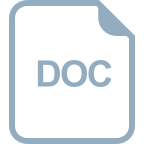
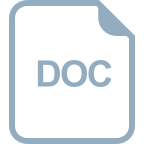
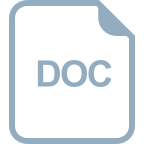













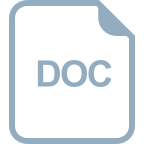
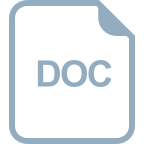
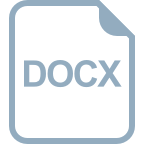