esp32 idf tcp server
时间: 2023-08-26 07:07:04 浏览: 115
The ESP32 IDF (Integrated Development Framework) can be used to create a TCP server on an ESP32 device. Here are the basic steps:
1. Include the necessary headers:
```
#include <stdio.h>
#include <string.h>
#include "freertos/FreeRTOS.h"
#include "freertos/task.h"
#include "esp_system.h"
#include "esp_wifi.h"
#include "esp_event.h"
#include "esp_log.h"
#include "nvs_flash.h"
#include "esp_netif.h"
#include "lwip/err.h"
#include "lwip/sys.h"
#include "lwip/netdb.h"
#include "lwip/api.h"
```
2. Define the server port number:
```
#define PORT_NUMBER 1234
```
3. Define the server task function:
```
void server_task(void *pvParameters)
{
struct netconn *conn, *newconn;
err_t err;
conn = netconn_new(NETCONN_TCP);
netconn_bind(conn, NULL, PORT_NUMBER);
netconn_listen(conn);
while(1)
{
err = netconn_accept(conn, &newconn);
if (err == ERR_OK)
{
char* buf = malloc(1024);
struct netbuf *recvbuf;
netconn_recv(newconn, &recvbuf);
netbuf_copy(recvbuf, buf, 1024);
netbuf_delete(recvbuf);
printf("Received data: %s\n", buf);
free(buf);
netconn_close(newconn);
netconn_delete(newconn);
}
}
}
```
4. Create the server task:
```
xTaskCreate(&server_task, "server_task", 4096, NULL, 5, NULL);
```
5. Initialize the ESP32 network interface:
```
esp_netif_init();
esp_event_loop_create_default();
esp_netif_create_default_wifi_sta();
wifi_init_config_t cfg = WIFI_INIT_CONFIG_DEFAULT();
esp_wifi_init(&cfg);
esp_wifi_set_storage(WIFI_STORAGE_RAM);
esp_wifi_set_mode(WIFI_MODE_STA);
esp_wifi_start();
```
6. Start the FreeRTOS scheduler:
```
vTaskStartScheduler();
```
This will create a TCP server that listens for incoming connections on port 1234. When a connection is established, the server will receive data from the client and print it to the console. Note that this code does not handle multiple connections or error conditions, and is intended as a starting point for further development.
阅读全文
相关推荐
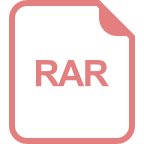















