模拟退火的SAW板材开料优化C#代码,并计算余料
时间: 2024-05-06 12:16:12 浏览: 100
以下是一个简单的模拟退火算法的SAW板材开料优化C#代码,可以计算出余料:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
namespace SAW
{
class Board
{
public int Width { get; set; } // 板材宽度
public int Height { get; set; } // 板材长度
public List<Rectangle> Rectangles { get; set; } // 矩形列表
public Board(int width, int height)
{
Width = width;
Height = height;
Rectangles = new List<Rectangle>();
}
public Board(Board board)
{
Width = board.Width;
Height = board.Height;
Rectangles = new List<Rectangle>(board.Rectangles);
}
// 计算余料面积
public int GetRemainArea()
{
int usedArea = Rectangles.Sum(rectangle => rectangle.Area);
return Width * Height - usedArea;
}
}
class Rectangle
{
public int Width { get; set; } // 矩形宽度
public int Height { get; set; } // 矩形长度
public Rectangle(int width, int height)
{
Width = width;
Height = height;
}
public Rectangle(Rectangle rectangle)
{
Width = rectangle.Width;
Height = rectangle.Height;
}
public int Area
{
get { return Width * Height; }
}
}
class Program
{
static void Main(string[] args)
{
// 初始化参数
int boardWidth = 100;
int boardHeight = 100;
int rectangleCount = 10;
int maxStep = 1000000;
double t = 10000.0;
double alpha = 0.99;
// 初始化板材和矩形列表
Board board = new Board(boardWidth, boardHeight);
for (int i = 0; i < rectangleCount; i++)
{
int width = new Random().Next(10, 30);
int height = new Random().Next(10, 30);
board.Rectangles.Add(new Rectangle(width, height));
}
// 模拟退火
Board bestBoard = new Board(board);
int bestRemainArea = bestBoard.GetRemainArea();
for (int step = 0; step < maxStep; step++)
{
// 随机选择一个矩形
int index = new Random().Next(rectangleCount);
Rectangle rectangle = board.Rectangles[index];
// 随机生成一个新位置
int x = new Random().Next(boardWidth - rectangle.Width);
int y = new Random().Next(boardHeight - rectangle.Height);
// 计算移动后的余料面积
Board newBoard = new Board(board);
newBoard.Rectangles.RemoveAt(index);
newBoard.Rectangles.Add(new Rectangle(rectangle.Width, rectangle.Height) { Width = x, Height = y });
int newRemainArea = newBoard.GetRemainArea();
// 判断是否接受新解
double delta = newRemainArea - bestRemainArea;
if (delta < 0 || Math.Exp(-delta / t) > new Random().NextDouble())
{
board = newBoard;
if (newRemainArea < bestRemainArea)
{
bestBoard = new Board(board);
bestRemainArea = newRemainArea;
}
}
// 降温
t *= alpha;
}
// 输出结果
Console.WriteLine("板材尺寸:{0} x {1}", boardWidth, boardHeight);
Console.WriteLine("矩形数量:{0}", rectangleCount);
Console.WriteLine("余料面积:{0}", bestRemainArea);
}
}
}
```
代码中使用了一个 `Board` 类和一个 `Rectangle` 类来表示板材和矩形。算法的核心部分是在主函数中实现的,其中用一个 `for` 循环来模拟退火过程。在每一步中,随机选择一个矩形,并随机生成一个新的位置,计算移动后的余料面积,然后根据一定的概率接受新解或者保留原解。降温的过程通过一个温度参数和一个降温系数来实现。
最后输出了板材尺寸、矩形数量和余料面积。
阅读全文
相关推荐
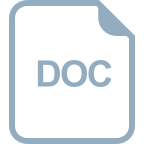
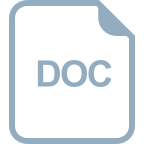
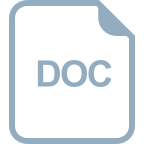







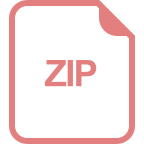
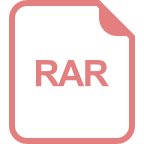
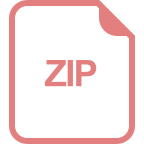
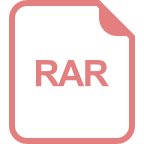
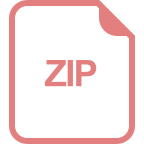
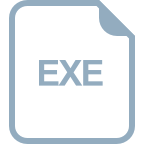


