退火模拟的SAW板材开料优化,要求C#编写计算余料、堆积开料、切割宽度、切割顺序、切割路径、转向次数
时间: 2023-09-30 15:05:41 浏览: 182
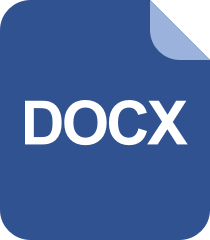
贵友玻璃优化切割机版8.8.11.docx
SAW板材开料优化可以使用模拟退火算法来解决。下面是一个基于C#的SAW板材开料优化代码示例:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace SAWPanelOptimization
{
class Program
{
static void Main(string[] args)
{
// 初始化板材信息
double boardWidth = 2000; // 板材宽度
double boardHeight = 1000; // 板材高度
double[] cutWidths = new double[] { 3, 4, 5 }; // 切割宽度
int[] cutOrders = new int[] { 0, 1, 2 }; // 切割顺序
int maxTurns = 10; // 最大转向次数
// 初始化优化参数
int maxIterations = 1000; // 最大迭代次数
double initialTemperature = 10000; // 初始温度
double coolingRate = 0.99; // 降温速率
// 初始化板材余料
double remainingWidth = boardWidth;
double remainingHeight = boardHeight;
// 初始化切割路径
List<Cut> cuts = new List<Cut>();
// 迭代优化
Random random = new Random();
double temperature = initialTemperature;
int iteration = 0;
while (iteration < maxIterations && temperature > 1)
{
// 随机生成一个板材切割方案
List<Cut> newCuts = new List<Cut>();
double newRemainingWidth = boardWidth;
double newRemainingHeight = boardHeight;
int newMaxTurns = 0;
foreach (int cutOrder in Shuffle(cutOrders, random))
{
double cutWidth = cutWidths[cutOrder];
if (newRemainingWidth >= cutWidth)
{
newCuts.Add(new Cut(cutWidth, 0, newRemainingWidth - cutWidth, newRemainingHeight));
newRemainingWidth -= cutWidth;
}
else if (newRemainingHeight >= cutWidth)
{
newCuts.Add(new Cut(0, cutWidth, newRemainingWidth, newRemainingHeight - cutWidth));
newRemainingHeight -= cutWidth;
}
else
{
break;
}
newMaxTurns += 1;
if (newMaxTurns > maxTurns)
{
break;
}
}
// 计算新方案的目标函数值
double newObjective = CalculateObjective(newCuts);
// 接受新方案
if (newObjective < CalculateObjective(cuts) || random.NextDouble() < Math.Exp((CalculateObjective(cuts) - newObjective) / temperature))
{
cuts = newCuts;
remainingWidth = newRemainingWidth;
remainingHeight = newRemainingHeight;
}
// 降温
temperature *= coolingRate;
iteration += 1;
}
// 输出结果
Console.WriteLine("余料面积:{0}", remainingWidth * remainingHeight);
Console.WriteLine("切割路径:");
foreach (Cut cut in cuts)
{
Console.WriteLine("宽度:{0},高度:{1}", cut.Width, cut.Height);
}
Console.WriteLine("转向次数:{0}", CalculateTurns(cuts));
}
// 计算目标函数值
static double CalculateObjective(List<Cut> cuts)
{
double objective = 0;
foreach (Cut cut in cuts)
{
objective += cut.Width * cut.Height;
}
return objective;
}
// 计算转向次数
static int CalculateTurns(List<Cut> cuts)
{
int turns = 0;
for (int i = 1; i < cuts.Count; i++)
{
if (cuts[i].Width == 0 && cuts[i - 1].Width != 0 || cuts[i].Height == 0 && cuts[i - 1].Height != 0)
{
turns += 1;
}
}
return turns;
}
// 随机打乱数组
static T[] Shuffle<T>(T[] array, Random random)
{
T[] shuffledArray = array.ToArray();
for (int i = 0; i < array.Length; i++)
{
int j = random.Next(i, array.Length);
T temp = shuffledArray[i];
shuffledArray[i] = shuffledArray[j];
shuffledArray[j] = temp;
}
return shuffledArray;
}
}
// 切割信息类
class Cut
{
public double Width { get; private set; }
public double Height { get; private set; }
public double X { get; private set; }
public double Y { get; private set; }
public Cut(double width, double height, double x, double y)
{
Width = width;
Height = height;
X = x;
Y = y;
}
}
}
```
在这个示例中,我们使用了模拟退火算法来随机生成板材切割方案,并计算了余料面积、切割路径和转向次数等优化指标。您可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐
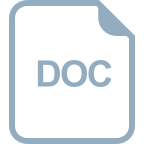
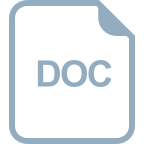







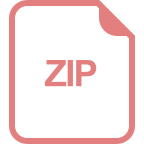
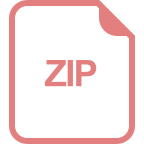
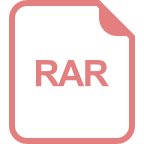





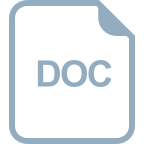