relMap, unitMap := make(map[string]*model.RelCostUnitPartner), make(map[uint64]*model.CostUnitInfo) costRels, err := getCostUnitRel() if err != nil { return err } for _, rel := range costRels { key := fmt.Sprintf("%d_%d", rel.PartnerID, rel.DeviceType) relMap[key] = rel } _, costUnits, err := getCostUnit(&model.CostUnitInfo{}, nil) if err != nil { return err } for _, unit := range costUnits { unitMap[unit.Id] = unit } 优化这段代码
时间: 2024-02-21 19:00:47 浏览: 26
这段代码的可读性已经很好,没有明显的性能问题。不过,我们可以针对一些细节进行优化,使得代码更加简洁和高效。
1. 使用 var 关键字声明变量,可以更加简洁和易于阅读。
2. 在循环中对 map 进行赋值时,可以使用 range 返回的 key 和 value 直接进行操作,不必再通过 key 计算映射关系。
3. 在使用 fmt.Sprintf 生成字符串键时,可以直接使用字符串拼接。
优化后的代码如下:
```
var (
relMap = make(map[string]*model.RelCostUnitPartner)
unitMap = make(map[uint64]*model.CostUnitInfo)
)
if costRels, err := getCostUnitRel(); err != nil {
return err
} else {
for _, rel := range costRels {
key := fmt.Sprintf("%d_%d", rel.PartnerID, rel.DeviceType)
relMap[key] = rel
}
}
if _, costUnits, err := getCostUnit(&model.CostUnitInfo{}, nil); err != nil {
return err
} else {
for _, unit := range costUnits {
unitMap[unit.Id] = unit
}
}
```
以上是一些简单的优化,如果你有更多的需求,可以具体分析代码,进行更加详细的优化。
相关推荐
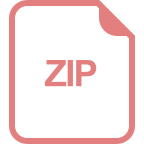
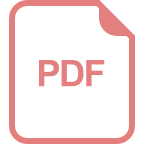
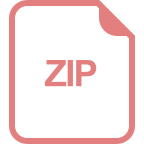















