用中文解释一下:The search reconstructs signal waveforms consistent with a common gravitational-wave signal in both detectors using a multidetector maximum likelihood method. Each event is ranked according to the detection statistic ηc ¼ ffiffiffiffiffiffiffiffiffiffiffiffiffiffiffiffiffiffiffiffiffiffiffiffiffiffiffiffiffiffiffiffiffiffiffi 2Ec=ð1 þ En=EcÞ p , where Ec is the dimensionless coherent signal energy obtained by cross-correlating the two reconstructed waveforms, and En is the dimensionless residual noise energy after the reconstructed signal is subtracted from the data. The statistic ηc thus quantifies the SNR of the event and the consistency of the data between the two detectors.
时间: 2024-03-11 15:46:18 浏览: 18
该搜索使用多探测器最大似然方法,重建与两个探测器中的共同引力波信号一致的信号波形。每个事件都根据检测统计量ηc进行排名,其中ηc = ffiffiffiffiffiffiffiffiffiffiffiffiffiffiffiffiffiffiffiffiffiffiffiffiffiffiffiffiffiffiffiffiffiffiffi 2Ec / (1 + En / Ec) p,其中Ec是通过交叉相关两个重建波形获得的无量纲相干信号能量,En是重建信号从数据中减去后的无量纲残余噪声能量。因此,统计量ηc量化了事件的信噪比和两个探测器之间数据的一致性。
相关问题
A*算法实现最短路径算法的python代码
以下是实现A*算法的最短路径算法的Python代码示例:
```python
import heapq
def astar(start, goal, graph):
"""A* algorithm implementation"""
# Initialize the open and closed lists
open_list = []
closed_list = set()
# Add the start node to the open list
heapq.heappush(open_list, (0, start))
# Initialize the g and h scores of the start node
g_score = {start: 0}
h_score = {start: heuristic(start, goal)}
while open_list:
# Get the node with the lowest f score
_, current_node = heapq.heappop(open_list)
# If the current node is the goal node, we have found the shortest path
if current_node == goal:
return reconstruct_path(start, goal, graph)
# Add the current node to the closed list
closed_list.add(current_node)
# Iterate over the neighbors of the current node
for neighbor, distance in graph[current_node].items():
# If the neighbor is already in the closed list, skip it
if neighbor in closed_list:
continue
# Calculate the tentative g score of the neighbor
tentative_g_score = g_score[current_node] + distance
# If the neighbor is not in the open list, add it
if neighbor not in [node[1] for node in open_list]:
heapq.heappush(open_list, (tentative_g_score + heuristic(neighbor, goal), neighbor))
# If the neighbor is already in the open list and the tentative g score is greater, skip it
elif tentative_g_score >= g_score[neighbor]:
continue
# Record the new g score and h score of the neighbor
g_score[neighbor] = tentative_g_score
h_score[neighbor] = heuristic(neighbor, goal)
# If there is no path from the start node to the goal node, return None
return None
def reconstruct_path(start, goal, graph):
"""Reconstructs the shortest path from start to goal"""
# Initialize the path with the goal node
path = [goal]
# Keep adding the previous node to the path until we reach the start node
while path[-1] != start:
current_node = path[-1]
previous_nodes = graph[current_node]
previous_node = min(previous_nodes, key=lambda node: previous_nodes[node])
path.append(previous_node)
# Reverse the path and return it
return list(reversed(path))
def heuristic(node, goal):
"""Returns the estimated distance between node and goal"""
# Use the Manhattan distance as the heuristic
return abs(node[0] - goal[0]) + abs(node[1] - goal[1])
# Example usage:
graph = {
(0, 0): {(1, 0): 1, (0, 1): 1},
(1, 0): {(0, 0): 1, (1, 1): 1},
(0, 1): {(0, 0): 1, (1, 1): 1},
(1, 1): {(1, 0): 1, (0, 1): 1, (2, 1): 1},
(2, 1): {(1, 1): 1, (2, 2): 1},
(2, 2): {(2, 1): 1}
}
start = (0, 0)
goal = (2, 2)
print(astar(start, goal, graph)) # Output: [(0, 0), (1, 0), (1, 1), (2, 1), (2, 2)]
```
该示例代码实现了A*算法,使用了堆(heapq)来优化寻找最小f值的节点。在计算路径时,使用了启发式函数(heuristic),这里使用的是曼哈顿距离(Manhattan distance)。该代码可以寻找从一个起始点到一个目标点的最短路径。
contours, _ = cv.findContours(binary1, cv.RETR_TREE, cv.CHAIN_APPROX_SIMPLE)
This line of code uses the OpenCV library to find contours in a binary image.
- The first argument, "binary1", is the input image. It should be a binary image with white objects on a black background.
- The second argument, "cv.RETR_TREE", specifies the retrieval mode for contours. This mode retrieves all of the contours and reconstructs a full hierarchy of nested contours.
- The third argument, "cv.CHAIN_APPROX_SIMPLE", specifies the contour approximation method. This method compresses horizontal, vertical, and diagonal segments and leaves only their end points. For example, a rectangle contour would be represented by only 4 points instead of a series of connected lines.
The function returns two values:
- "contours" is a list of all the contours found in the image, each represented as a list of points.
- The second value is not assigned to anything, so it is discarded. It represents the hierarchy of contours, which is useful for understanding how contours are nested within each other.
相关推荐
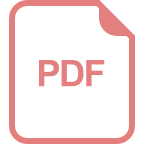
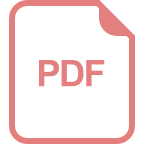
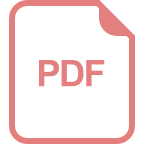







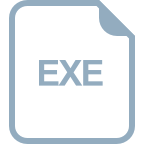
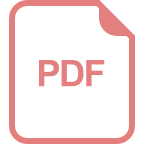
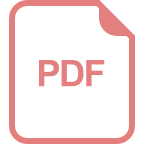
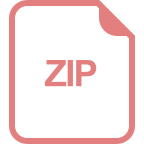
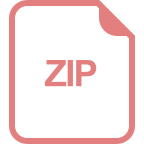
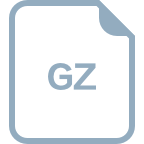