移位寄存器verilog
时间: 2023-06-22 13:22:35 浏览: 66
移位寄存器是一种常用的数字电路元件,可以实现对二进制数据进行移位操作。在 Verilog 中,可以使用以下语法来实现一个 4 位移位寄存器:
```
module shift_register(
input clk,
input reset,
input shift_in,
output reg [3:0] shift_out
);
always @(posedge clk, posedge reset) begin
if (reset) begin
shift_out <= 4'b0;
end else begin
shift_out <= {shift_out[2:0], shift_in};
end
end
endmodule
```
这里使用了 always 语句来描述移位寄存器的行为。每当时钟信号 clk 上升沿到来时,会执行 always 语句中的代码块。在代码块中,首先判断 reset 信号是否为高电平。若为高电平,则将 shift_out 寄存器清零;否则,将 shift_out 中的前三位向左移动一位,并将 shift_in 的值存入 shift_out 的最低位。
通过这个 Verilog 代码,我们可以实现一个带有复位功能的 4 位移位寄存器。
相关问题
移位寄存器 verilog
移位寄存器(Shift Register)是一种时序电路,可以将数据按照一定的规则进行移位操作。在Verilog中,可以通过以下代码实现一个4位移位寄存器:
module shift_register(
input clk, // 时钟输入
input [3:0] data_in, // 数据输入
input shift_en, // 移位使能信号
output reg [3:0] data_out // 数据输出
);
always @(posedge clk) begin
if(shift_en) begin
data_out <= {data_out[2:0], data_in}; // 右移一位,并将输入数据放到最左边
end
end
endmodule
其中,data_out表示移位寄存器当前的值,shift_en为使能信号,当它为1时,移位寄存器开始工作,执行一次右移操作,并将data_in放在最左边。
移位寄存器verilog循环
Verilog 中的移位寄存器可以通过循环移位操作实现循环移位的功能。下面是一个简单的 4 位循环移位寄存器的 Verilog 代码:
```
module circular_shift_reg(input clk, rst, shift, data_in, output reg [3:0] data_out);
always @(posedge clk, posedge rst)
if (rst)
data_out <= 4'b0000;
else if (shift)
data_out <= {data_out[2:0], data_out[3]};
else
data_out <= data_out;
endmodule
```
该代码与前面的移位寄存器代码非常相似,区别在于移位操作。当 `shift` 为高电平时,`data_out` 向左移动一位,并将最高位放入最低位;否则,`data_out` 不变。这样,就实现了循环移位的功能。
使用该代码,可以实现一个简单的 4 位循环移位寄存器。
相关推荐









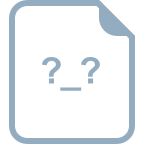
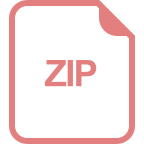
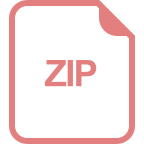
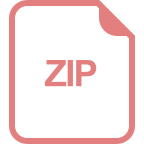