分别写出在散列表中插入和删除关键字为k的一个记录的算法,设散列函数为h,解决冲突的方法为链地址法。
时间: 2023-04-20 19:02:03 浏览: 319
在散列表中插入关键字为k的记录的算法:
1. 根据散列函数h计算出关键字k的散列地址h(k);
2. 在散列表中查找散列地址h(k)对应的链表;
3. 如果链表为空,则在该位置插入一个新节点,节点的关键字为k;
4. 如果链表不为空,则遍历链表,查找是否已经存在关键字为k的节点;
5. 如果已经存在,则更新该节点的值;
6. 如果不存在,则在链表的末尾插入一个新节点,节点的关键字为k。
在散列表中删除关键字为k的记录的算法:
1. 根据散列函数h计算出关键字k的散列地址h(k);
2. 在散列表中查找散列地址h(k)对应的链表;
3. 如果链表为空,则直接返回,因为不存在关键字为k的节点;
4. 如果链表不为空,则遍历链表,查找是否存在关键字为k的节点;
5. 如果存在,则删除该节点;
6. 如果不存在,则直接返回,因为不存在关键字为k的节点。
相关问题
分别写出在散列表中插入和删除关键字为K的一个记录的算法,设散列函数为H,解决冲突的方法为链地址法。
散列表中插入关键字为K的记录的算法:
1. 计算关键字K的散列地址h = H(K)
2. 在散列表中查找散列地址为h的链表
3. 如果链表为空,创建一个新节点,将K插入到链表中,返回成功
4. 如果链表不为空,遍历链表,查找是否存在关键字为K的记录
5. 如果存在,返回失败
6. 如果不存在,创建一个新节点,将K插入到链表头部,返回成功
散列表中删除关键字为K的记录的算法:
1. 计算关键字K的散列地址h = H(K)
2. 在散列表中查找散列地址为h的链表
3. 如果链表为空,返回失败
4. 如果链表不为空,遍历链表,查找是否存在关键字为K的记录
5. 如果不存在,返回失败
6. 如果存在,删除该节点,返回成功
编写代码实现分别写出在散列表中插入和删除关键字为K的一个记录的算法,设散列函数为H,解决冲突的方法为链地址法。
以下是Python实现的代码:
```python
class Node:
def __init__(self, key=None, value=None, next=None):
self.key = key
self.value = value
self.next = next
class HashTable:
def __init__(self, size=100):
self.size = size
self.table = [None] * size
def hash_func(self, key):
return hash(key) % self.size
def insert(self, key, value):
h = self.hash_func(key)
if self.table[h] is None:
self.table[h] = Node(key, value)
else:
node = self.table[h]
while node.next:
if node.key == key:
return False
node = node.next
if node.key == key:
return False
else:
node.next = Node(key, value)
return True
def delete(self, key):
h = self.hash_func(key)
if self.table[h] is None:
return False
elif self.table[h].key == key:
self.table[h] = self.table[h].next
else:
node = self.table[h]
while node.next:
if node.next.key == key:
node.next = node.next.next
return True
node = node.next
return False
```
这里使用了链表来解决冲突,如果散列表中的某个位置已经有了元素,就在链表的末尾插入新元素。在删除元素时,需要遍历链表来找到要删除的元素。
相关推荐
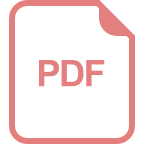
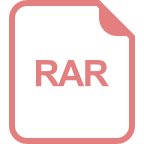
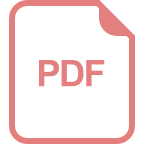












