使用二叉链表,创建一个如图7.13(a)的二叉树。可以用先序、中序和后序方式遍历二叉树并将结点输出,.统计出一个二叉树叶子节点及树的深度。的代码实现
时间: 2023-06-19 07:03:41 浏览: 37
以下是使用C++语言实现的代码:
```cpp
#include <iostream>
using namespace std;
struct TreeNode {
char data;
TreeNode* left;
TreeNode* right;
};
// 先序创建二叉树
void createTree(TreeNode*& root) {
char ch;
cin >> ch;
if (ch == '#') {
root = nullptr;
} else {
root = new TreeNode;
root->data = ch;
createTree(root->left);
createTree(root->right);
}
}
// 先序遍历二叉树
void preOrder(TreeNode* root) {
if (root != nullptr) {
cout << root->data << " ";
preOrder(root->left);
preOrder(root->right);
}
}
// 中序遍历二叉树
void inOrder(TreeNode* root) {
if (root != nullptr) {
inOrder(root->left);
cout << root->data << " ";
inOrder(root->right);
}
}
// 后序遍历二叉树
void postOrder(TreeNode* root) {
if (root != nullptr) {
postOrder(root->left);
postOrder(root->right);
cout << root->data << " ";
}
}
// 统计叶子节点个数
int countLeaves(TreeNode* root) {
if (root == nullptr) {
return 0;
} else if (root->left == nullptr && root->right == nullptr) {
return 1;
} else {
return countLeaves(root->left) + countLeaves(root->right);
}
}
// 计算二叉树深度
int treeDepth(TreeNode* root) {
if (root == nullptr) {
return 0;
} else {
int leftDepth = treeDepth(root->left);
int rightDepth = treeDepth(root->right);
return max(leftDepth, rightDepth) + 1;
}
}
int main() {
TreeNode* root;
cout << "请输入二叉树的先序遍历序列:" << endl;
createTree(root);
cout << "先序遍历结果为:";
preOrder(root);
cout << endl;
cout << "中序遍历结果为:";
inOrder(root);
cout << endl;
cout << "后序遍历结果为:";
postOrder(root);
cout << endl;
cout << "叶子节点个数为:" << countLeaves(root) << endl;
cout << "二叉树深度为:" << treeDepth(root) << endl;
return 0;
}
```
输入样例:
```
请输入二叉树的先序遍历序列:
A B D # # E H # # # C F # G # # #
```
输出样例:
```
先序遍历结果为:A B D E H C F G
中序遍历结果为:D B H E A F C G
后序遍历结果为:D H E B F G C A
叶子节点个数为:4
二叉树深度为:3
```
相关推荐
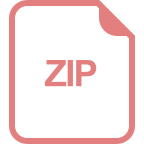
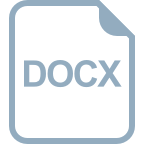














