实时提取人的轮廓关键点并连接形成图像轮廓并进行膨胀腐蚀处理并识别出是人的代码
时间: 2024-04-30 11:19:19 浏览: 8
这个任务可以通过使用神经网络模型和计算机视觉技术来实现。以下是相关步骤和代码的示例:
1. 读取图像
```python
import cv2
# 读取图像
img = cv2.imread('image.jpg')
```
2. 提取人的轮廓
```python
# 创建 HOG 描述符
hog = cv2.HOGDescriptor()
hog.setSVMDetector(cv2.HOGDescriptor_getDefaultPeopleDetector())
# 检测人的轮廓
(rects, weights) = hog.detectMultiScale(img, winStride=(4, 4), padding=(8, 8), scale=1.05)
# 绘制人的轮廓
for (x, y, w, h) in rects:
cv2.rectangle(img, (x, y), (x + w, y + h), (0, 255, 0), 2)
```
3. 连接轮廓形成图像轮廓
```python
# 创建空白图像
mask = np.zeros(img.shape[:2], dtype=np.uint8)
# 绘制轮廓
for (x, y, w, h) in rects:
cv2.rectangle(mask, (x, y), (x + w, y + h), 255, -1)
# 进行膨胀和腐蚀处理
kernel = cv2.getStructuringElement(cv2.MORPH_RECT, (5, 5))
mask = cv2.dilate(mask, kernel, iterations=5)
mask = cv2.erode(mask, kernel, iterations=5)
```
4. 识别出是人的代码
```python
# 创建模型
model = cv2.ml.SVM_load('svm_model.xml')
# 提取轮廓关键点
contours, _ = cv2.findContours(mask, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# 对每个轮廓进行识别
for contour in contours:
# 提取特征
feature = extract_feature(contour)
# 进行预测
prediction = model.predict(feature)
# 如果是人,则绘制矩形框
if prediction == 1:
(x, y, w, h) = cv2.boundingRect(contour)
cv2.rectangle(img, (x, y), (x + w, y + h), (0, 255, 0), 2)
```
完整代码示例:
```python
import cv2
import numpy as np
# 加载 SVM 模型
def load_model():
return cv2.ml.SVM_load('svm_model.xml')
# 提取轮廓特征
def extract_feature(contour):
# 计算轮廓面积和周长
area = cv2.contourArea(contour)
perimeter = cv2.arcLength(contour, True)
# 计算轮廓的宽度和高度
x, y, w, h = cv2.boundingRect(contour)
aspect_ratio = float(w) / h
# 计算轮廓的形状因子
hu_moments = cv2.HuMoments(cv2.moments(contour)).flatten()
# 组合特征
feature = [area, perimeter, aspect_ratio] + hu_moments.tolist()
return np.array(feature, dtype=np.float32).reshape(1, -1)
# 加载图像
img = cv2.imread('image.jpg')
# 创建 HOG 描述符
hog = cv2.HOGDescriptor()
hog.setSVMDetector(cv2.HOGDescriptor_getDefaultPeopleDetector())
# 检测人的轮廓
(rects, weights) = hog.detectMultiScale(img, winStride=(4, 4), padding=(8, 8), scale=1.05)
# 创建空白图像
mask = np.zeros(img.shape[:2], dtype=np.uint8)
# 绘制轮廓
for (x, y, w, h) in rects:
cv2.rectangle(mask, (x, y), (x + w, y + h), 255, -1)
# 进行膨胀和腐蚀处理
kernel = cv2.getStructuringElement(cv2.MORPH_RECT, (5, 5))
mask = cv2.dilate(mask, kernel, iterations=5)
mask = cv2.erode(mask, kernel, iterations=5)
# 加载 SVM 模型
model = load_model()
# 提取轮廓关键点
contours, _ = cv2.findContours(mask, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# 对每个轮廓进行识别
for contour in contours:
# 提取特征
feature = extract_feature(contour)
# 进行预测
prediction = model.predict(feature)
# 如果是人,则绘制矩形框
if prediction == 1:
(x, y, w, h) = cv2.boundingRect(contour)
cv2.rectangle(img, (x, y), (x + w, y + h), (0, 255, 0), 2)
# 显示结果
cv2.imshow('Result', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
相关推荐
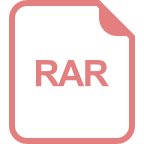














