定义一个基类Shape,在此基础上派生出Rectangle和Circle类,二者都有Getarea()计算对象面积
时间: 2024-05-10 12:18:43 浏览: 19
下面是一个示例代码,实现了Shape基类以及Rectangle和Circle派生类,并且每个类都有GetArea()函数来计算对象面积:
```c++
#include <iostream>
using namespace std;
class Shape
{
public:
virtual double GetArea() = 0;
};
class Rectangle : public Shape
{
private:
double length;
double width;
public:
Rectangle(double l, double w) : length(l), width(w) {}
double GetArea() { return length * width; }
};
class Circle : public Shape
{
private:
double radius;
public:
Circle(double r) : radius(r) {}
double GetArea() { return 3.1415926 * radius * radius; }
};
int main()
{
Shape* shape1 = new Rectangle(5, 10);
Shape* shape2 = new Circle(3);
cout << "Rectangle area: " << shape1->GetArea() << endl;
cout << "Circle area: " << shape2->GetArea() << endl;
delete shape1;
delete shape2;
return 0;
}
```
在上面的代码中,Shape是一个纯虚基类,它提供了一个GetArea()函数但没有实现。Rectangle和Circle派生类都实现了这个函数,并且在main函数里我们创建了一个Rectangle对象和一个Circle对象,并分别调用它们的GetArea()函数来计算它们的面积。注意,我们使用了Shape指针来指向Rectangle和Circle对象,这是因为Shape是一个基类,它的指针可以指向任何派生类对象。
相关推荐
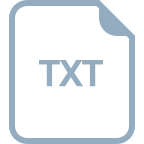
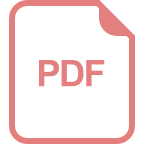
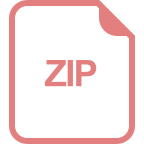













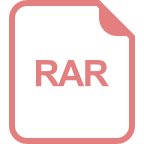
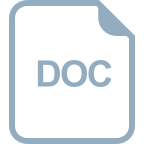