devcpp实现定义一个基类Shape,在此基础上派生出Rectangle和Circle类,二者都有GetArea()计算对象的面积。
时间: 2024-05-04 21:21:46 浏览: 102
可以按照以下步骤来实现:
1. 定义基类Shape,声明GetArea()函数作为接口。
```cpp
class Shape {
public:
virtual double GetArea() = 0; // 纯虚函数,作为接口
};
```
2. 派生出Rectangle类和Circle类,分别实现GetArea()函数。
```cpp
class Rectangle : public Shape {
private:
double width;
double height;
public:
Rectangle(double w, double h) : width(w), height(h) {}
double GetArea() override {
return width * height;
}
};
class Circle : public Shape {
private:
double radius;
public:
Circle(double r) : radius(r) {}
double GetArea() override {
return 3.14 * radius * radius;
}
};
```
3. 在main函数中创建对象并调用GetArea()函数计算面积。
```cpp
int main() {
Rectangle rect(3.0, 4.0);
Circle circle(5.0);
cout << "Rectangle area: " << rect.GetArea() << endl;
cout << "Circle area: " << circle.GetArea() << endl;
return 0;
}
```
这样就可以实现一个简单的面向对象的计算面积程序。
阅读全文
相关推荐
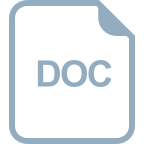
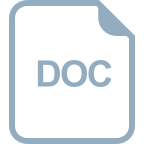
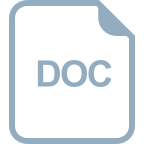















