声明一个基类Shape,在此基础上派生Rectangle和Circle,二者都有GetArea(),Retangle类派生了Square类,也有GetArea()函数计算对象的面积,编写程序测试。注意同名隐藏和类型兼容两种规则的体现,实现机制,可以使用派生类的对象调用同名函数,使用基类指针调用同名函数。
时间: 2024-02-21 12:02:27 浏览: 141
下面是一个实现上述题目要求的C++代码示例:
```c++
#include<iostream>
using namespace std;
class Shape {
public:
virtual double GetArea() { return 0; } //虚函数,计算面积
};
class Rectangle : public Shape {
protected:
double width;
double height;
public:
Rectangle(double w, double h) : width(w), height(h) {}
virtual double GetArea() { return width * height; } //重写虚函数
};
class Circle : public Shape {
protected:
double radius;
public:
Circle(double r) : radius(r) {}
virtual double GetArea() { return 3.14 * radius * radius; } //重写虚函数
};
class Square : public Rectangle {
public:
Square(double l) : Rectangle(l, l) {} //利用Rectangle的构造函数初始化
virtual double GetArea() { return width * width; } //重写虚函数
};
int main() {
Shape* shape1 = new Rectangle(3, 4); //用基类指针指向派生类对象
Shape* shape2 = new Circle(5);
Shape* shape3 = new Square(6);
cout << "Rectangle area is: " << shape1->GetArea() << endl; //使用基类指针调用同名函数
cout << "Circle area is: " << shape2->GetArea() << endl;
cout << "Square area is: " << shape3->GetArea() << endl;
Rectangle* rect1 = new Rectangle(4, 5);
Square* square1 = new Square(6);
cout << "Rectangle area is: " << rect1->GetArea() << endl; //使用派生类对象调用同名函数
cout << "Square area is: " << square1->GetArea() << endl;
delete shape1;
delete shape2;
delete shape3;
delete rect1;
delete square1;
return 0;
}
```
在上述代码中,我们首先定义了一个基类Shape,其中包含一个虚函数GetArea(),用于计算派生类对象(如Rectangle、Circle、Square)的面积。然后我们分别定义了Rectangle、Circle、Square三个派生类,其中Rectangle和Circle直接继承自Shape,而Square则继承自Rectangle。在每个派生类中,我们都重写了GetArea()方法,以计算对应的面积。
在main函数中,我们创建了三个基类指针shape1、shape2、shape3,分别指向一个Rectangle、一个Circle、一个Square对象。这里需要注意,我们可以使用基类指针调用同名函数,这是因为虚函数和动态绑定的机制,能够在运行时根据派生类对象的实际类型调用正确的函数。
我们还创建了两个派生类对象rect1和square1,使用这两个对象调用GetArea()方法时,同样会调用各自类中的重写函数。
最后,记得释放动态分配的对象,以避免内存泄漏。
阅读全文
相关推荐
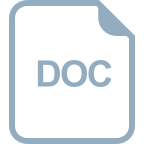
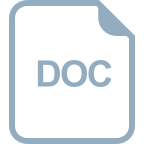
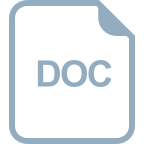













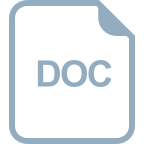
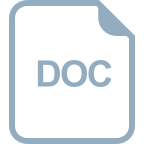
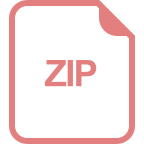