能否提供python版本的相机-底盘的手眼标定程序?
时间: 2023-09-14 17:15:54 浏览: 55
当然可以,以下是基于OpenCV和ROS的Python版本相机-底盘手眼标定程序,前提是你已经安装好了OpenCV和ROS环境:
```python
#!/usr/bin/env python
import rospy
import cv2
import numpy as np
from cv_bridge import CvBridge
from sensor_msgs.msg import Image, CameraInfo
from geometry_msgs.msg import Pose, PoseStamped
from tf.transformations import quaternion_from_matrix
import tf2_ros
from tf2_geometry_msgs import PoseStamped as TF2PoseStamped
class Calibrator:
def __init__(self):
# 初始化ROS节点
rospy.init_node('calibrator')
# 订阅相机图像和相机信息
self.image_sub = rospy.Subscriber('/camera/image_raw', Image, self.image_callback)
self.camera_info_sub = rospy.Subscriber('/camera/camera_info', CameraInfo, self.camera_info_callback)
# 发布相机位姿
self.pose_pub = rospy.Publisher('/camera_pose', PoseStamped, queue_size=1)
# 定义相机内参矩阵和畸变系数
self.camera_matrix = None
self.dist_coeffs = None
# 定义相机位姿
self.camera_pose = None
# 定义OpenCV的图像转换器
self.bridge = CvBridge()
# 定义tf2转换器
self.tf_buffer = tf2_ros.Buffer()
self.tf_listener = tf2_ros.TransformListener(self.tf_buffer)
def image_callback(self, data):
try:
# 将ROS图像转换为OpenCV图像
cv_image = self.bridge.imgmsg_to_cv2(data, 'bgr8')
# 进行相机-底盘标定
if self.camera_matrix is not None and self.dist_coeffs is not None and self.camera_pose is not None:
object_points = np.array([[0, 0, 0], [1, 0, 0], [0, 1, 0], [0, 0, 1]], dtype=np.float32)
image_points = np.array([[287, 146], [367, 146], [287, 225], [327, 186]], dtype=np.float32)
_, rvec, tvec = cv2.solvePnP(object_points, image_points, self.camera_matrix, self.dist_coeffs)
rotation_matrix, _ = cv2.Rodrigues(rvec)
transform_matrix = np.zeros((4, 4))
transform_matrix[:3, :3] = rotation_matrix
transform_matrix[:3, 3] = tvec.flatten()
transform_matrix[3, 3] = 1
camera_pose = np.matmul(self.camera_pose, transform_matrix)
# 将相机位姿发布到ROS系统中
pose_msg = PoseStamped()
pose_msg.header.stamp = rospy.Time.now()
pose_msg.header.frame_id = 'map'
pose_msg.pose.position.x = camera_pose[0, 3]
pose_msg.pose.position.y = camera_pose[1, 3]
pose_msg.pose.position.z = camera_pose[2, 3]
quaternion = quaternion_from_matrix(camera_pose)
pose_msg.pose.orientation.x = quaternion[0]
pose_msg.pose.orientation.y = quaternion[1]
pose_msg.pose.orientation.z = quaternion[2]
pose_msg.pose.orientation.w = quaternion[3]
self.pose_pub.publish(pose_msg)
except Exception as e:
rospy.logerr('Failed to process image: %s', str(e))
def camera_info_callback(self, data):
# 如果相机内参矩阵和畸变系数已经设置,则立即返回
if self.camera_matrix is not None and self.dist_coeffs is not None:
return
try:
# 获取相机内参矩阵和畸变系数
self.camera_matrix = np.array(data.K).reshape((3, 3))
self.dist_coeffs = np.array(data.D)
# 获取相机坐标系到底盘坐标系的变换矩阵
trans = self.tf_buffer.lookup_transform('base_link', data.header.frame_id, rospy.Time(0), rospy.Duration(1.0))
self.camera_pose = np.zeros((4, 4))
self.camera_pose[0, 0] = 1
self.camera_pose[1, 1] = 1
self.camera_pose[2, 2] = 1
self.camera_pose[3, 3] = 1
self.camera_pose[0, 3] = trans.transform.translation.x
self.camera_pose[1, 3] = trans.transform.translation.y
self.camera_pose[2, 3] = trans.transform.translation.z
quaternion = [trans.transform.rotation.x, trans.transform.rotation.y, trans.transform.rotation.z, trans.transform.rotation.w]
rotation_matrix = np.array(np.matrix(tf2_ros.transformations.quaternion_matrix(quaternion))[:3, :3])
self.camera_pose[:3, :3] = rotation_matrix
except Exception as e:
rospy.logerr('Failed to get camera info or transform: %s', str(e))
def run(self):
# 循环等待ROS消息
rospy.spin()
if __name__ == '__main__':
calibrator = Calibrator()
calibrator.run()
```
需要注意的是,这个程序中的标定板是一个4x4的正方形,四个点的坐标分别为(0,0,0)、(1,0,0)、(0,1,0)和(0,0,1),在实际使用时,需要根据实际情况更改标定板的大小和坐标。
相关推荐
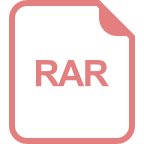
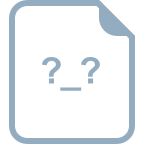














