多尺度图像融合python
时间: 2023-05-13 13:01:21 浏览: 137
多尺度图像融合是指将具有不同分辨率的多个图像融合为一个高质量且具有更广广面的信息的图像。Python语言对于多尺度图像融合具有多种实现方式,可以基于OpenCV等图像处理库进行实现。
一种实现方式是采用拉普拉斯金字塔进行图像融合。首先对于两张待融合的图像进行高斯金字塔拆分,得到一系列不同分辨率的图像,再根据拉普拉斯金字塔的原理进行图像融合,得到最终结果图。具体步骤为:先对两张图像进行高斯滤波,得到具有不同分辨率的图像,在进行相减得到差值图;将差值图和高斯金字塔的上一层图像相加得到新的差值图,并重复上述步骤直到高斯金字塔的最高层;最后对所有各层差值图进行融合得到最终图像。
另一种实现方式是采用小波变换进行图像融合。小波变换可以将一幅图像分解为多个子带图像,再将不同子带图像进行融合得到最终结果图。具体步骤为:对两张待融合的图像进行小波分解得到各个子带图像,在对子带图像进行加权融合得到各个融合子带图像;将各个融合子带图像进行小波重构得到最终结果图。
以上两种实现方式都可以实现多尺度图像融合,可以根据具体情况选择不同的实现方式。在Python语言中,借助OpenCV等图像处理库的函数,能够更加方便和高效地实现多尺度图像融合。
相关问题
python多尺度图像融合
多尺度融合是一种图像处理技术,通常用于增强图像的边缘和细节。在Python中,可以使用OpenCV库来实现多尺度融合。下面是一个简单的多尺度融合的Python代码示例:
```python
import cv2
# 读取图像
img = cv2.imread('image.jpg')
# 生成图像金字塔
pyramid = []
temp = img.copy()
pyramid.append(temp)
for i in range(6):
temp = cv2.pyrDown(temp)
pyramid.append(temp)
# 从金字塔顶部开始迭代融合
result = pyramid[5]
for i in range(5, 0, -1):
result = cv2.addWeighted(pyramid[i-1], 1.5, result, -0.5, 0)
# 显示融合后的图像
cv2.imshow('Multi-Scale Fusion', result)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
python基于小波变换的图像融合
Python基于小波变换的图像融合是一种数字图像处理的方法,通过将不同的图像融合在一起,来得到更高质量的图像。小波变换是一种时频分析方法,它可以将信号分解成不同尺度的频率组成,并且保留图像的空间和时间域特征。
图像融合的过程可以分为以下几步:首先对两幅图像进行小波变换,得到低频分量和高频分量,然后对低频分量进行图像平滑,消除噪声和细节信息;对高频分量进行图像增强,增强图像中的细节和轮廓信息。最后将两幅图像的低频分量和高频分量合并起来,得到一幅更加清晰明亮的图像。
Python提供了多种小波变换库,如pywt,scipy等,通过这些库可以方便地实现小波变换,并且进行图像融合。同时还可以调节平滑和增强的参数,得到更好的融合效果。
小波变换的图像融合在很多领域都有应用,如医学图像处理、遥感图像处理等。通过不同的融合方式,可以得到不同的效果,有助于更好地解决实际问题。
相关推荐
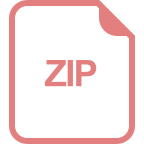
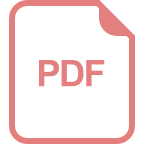












