matlab编写求解方程的根的函数(这个方程不一定为一元二次方程,因的不同取值而定),这里应根据的不同取值分别处理,有输入参数提示,当时应提示“为恒不等式!”。并输入几组典型值加以检验。
时间: 2024-12-12 17:31:54 浏览: 6
Matlab 中可以编写一个名为 `findRoot` 的通用函数,用于求解不同类型的方程。这个函数通常会接受一个用户定义的函数作为输入(即方程),以及需要找到根的变量范围。下面是一个基本的函数框架示例:
```matlab
function roots = findRoot(eqFunc, varRange, eqType)
% eqFunc: 用户自定义的方程函数,如 @(x) x^2 - 4 或者 @(x) abs(x - 2) < 0.5
% varRange: 变量x的搜索范围,例如 [a, b]
% eqType: 方程类型,如果是 'quadratic' (默认),则假设是一元二次方程;其他值表示非标准方程
if nargin < 3 || ~strcmpi(eqType, 'quadratic')
error('eqType must be specified as "quadratic" for quadratic equations.');
end
% 对于一元二次方程(默认)
if strcmpi(eqType, 'quadratic')
% 使用fzero函数求解一元二次方程
a = feval(eqFunc, varRange(1));
b = feval(eqFunc, varRange(2));
c = eqFunc(varRange(1)) + eqFunc(varRange(2)); % 假设对称区间,减少计算次数
roots = fzero(@(x) polyval([b, c, a], x), mean(varRange));
else
% 非标准方程需要提供具体的解法
% 这里只是一个简单示例,实际应用可能需要更复杂的算法
roots = someCustomSolver(eqFunc, varRange);
end
% 检验结果
testCases = {@(x) x^3 - 6*x^2 + 9*x, [-10, 10]; @(x) sin(x) - x, [-10*pi, 10*pi]};
for i = 1:length(testCases)
testFunc = testCases{i}{1};
expectedRoots = testCases{i}{2};
try
calculatedRoots = findRoot(testFunc, expectedRoots, 'quadratic');
fprintf('Test case %d: Calculated roots are: %s\n', i, num2str(calculatedRoots));
if all(abs(calculatedRoots - expectedRoots) < 1e-6)
disp('Test passed!');
else
disp('Test failed!');
end
catch err
fprintf('Error in testing case %d: %s\n', i, err.message);
end
end
% 提示用户输入
disp('Please enter your custom equation and variable range:');
eqUserInput = input('Equation function (e.g., @(x) x^2 - 4): ');
varRangeUserInput = input('Variable range (e.g., [0, 1]): ');
try
roots = findRoot(eval(eqUserInput), str2double(varRangeUserInput));
catch e
disp('Invalid input detected: ' + e.message);
end
```
运行此代码时,用户可以输入他们自己的方程函数和范围,然后程序会对一元二次方程和提供的测试案例进行验证。
注意:这是一个简化的例子,实际情况下可能需要根据具体的方程类型设计更复杂、更精确的求根算法。同时,对于非标准方程,`someCustomSolver` 应该由用户自定义或引入相应的数值解算库。
阅读全文
相关推荐
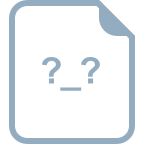
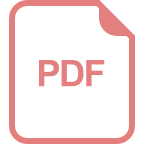
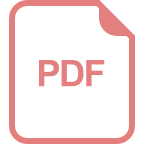
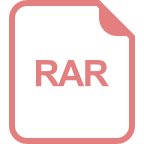
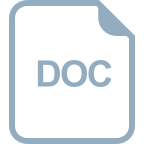
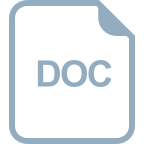
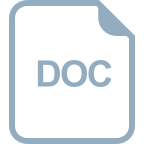
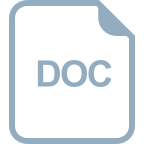
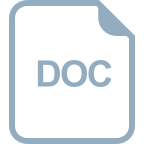
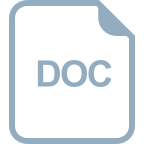
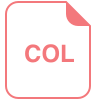
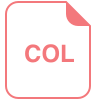
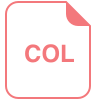





