编写一个程序,对于任意输入的两个整数,求商和余数。
时间: 2024-10-16 11:12:12 浏览: 35
编写一个简单的Python程序来计算任意两个整数相除的商和余数,可以使用取模运算符`%`来获取余数。以下是程序示例:
```python
def calculate_division(dividend, divisor):
"""
计算两个整数的商和余数
:param dividend: 被除数
:param divisor: 除数
:return: 商 (int) 和余数 (int)
"""
quotient = dividend // divisor # 商
remainder = dividend % divisor # 余数
return quotient, remainder
# 示例输入
dividend = int(input("请输入被除数:"))
divisor = int(input("请输入除数:"))
# 计算并打印结果
result = calculate_division(dividend, divisor)
print(f"商:{result[0]}, 余数:{result[1]}")
#
相关问题
用Dev c++编写一个程序,对于任意输入的两个整数,求商和余数。
在Dev C++中,你可以使用`%`操作符来获取两个整数相除的余数,并通过算术运算来得到商。下面是一个简单的示例程序:
```cpp
#include <iostream>
int main() {
int dividend; // 被除数
int divisor; // 除数
int quotient; // 商
int remainder; // 余数
// 输入两个整数
std::cout << "请输入第一个整数 (被除数): ";
std::cin >> dividend;
std::cout << "请输入第二个整数 (除数): ";
std::cin >> divisor;
// 计算商和余数
quotient = dividend / divisor;
remainder = dividend % divisor;
// 输出结果
std::cout << "商是: " << quotient << "\n";
std::cout << "余数是: " << remainder << "\n";
return 0;
}
```
在这个程序中,用户会提示输入两个整数,然后计算它们的商和余数并显示出来。
编写一个程序,对于任意输入的整数a和b,求商和余数
当你想编写一个程序来计算两个整数a和b之间的商(quotient)和余数(remainder),你可以使用C++的`divmod`运算符或者手动进行除法运算并取余。这里是一个简单的例子:
```cpp
#include <iostream>
int main() {
int a, b;
std::cout << "Enter two integers (a and b): ";
std::cin >> a >> b;
// 使用 divmod 运算符同时获取商和余数
int quotient, remainder;
std::tie(quotient, remainder) = div(a, b);
std::cout << "Quotient: " << quotient << "\n";
std::cout << "Remainder: " << remainder << "\n";
return 0;
}
```
在这个程序中,我们首先从用户那里获取两个整数a和b,然后用`std::tie`和`div`函数来计算商和余数。`div`返回一个pair类型的结果,包含了商和余数。
如果要手动进行除法和取余操作,可以这样做:
```cpp
#include <iostream>
int main() {
int a, b;
std::cout << "Enter two integers (a and b): ";
std::cin >> a >> b;
int quotient = a / b; // 商
int remainder = a % b; // 余数
std::cout << "Quotient: " << quotient << "\n";
std::cout << "Remainder: " << remainder << "\n";
return 0;
}
```
阅读全文
相关推荐
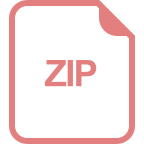
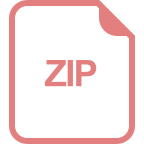
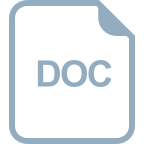

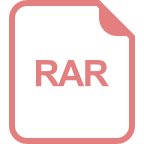











