用c实现将unsigned char数组里的数据保存为jpg文件
时间: 2024-01-21 08:18:31 浏览: 205
要将unsigned char数组保存为jpg文件,需要使用libjpeg库。以下是一个简单的示例代码:
```c
#include <stdio.h>
#include <jpeglib.h>
void save_jpg(unsigned char *image, int width, int height, char *filename) {
struct jpeg_compress_struct cinfo;
struct jpeg_error_mgr jerr;
FILE *outfile = fopen(filename, "wb");
if (!outfile) {
fprintf(stderr, "Error: can't open %s for writing\n", filename);
return;
}
cinfo.err = jpeg_std_error(&jerr);
jpeg_create_compress(&cinfo);
jpeg_stdio_dest(&cinfo, outfile);
cinfo.image_width = width;
cinfo.image_height = height;
cinfo.input_components = 3;
cinfo.in_color_space = JCS_RGB;
jpeg_set_defaults(&cinfo);
jpeg_start_compress(&cinfo, TRUE);
JSAMPROW row_pointer[1];
int row_stride = width * 3;
while (cinfo.next_scanline < cinfo.image_height) {
row_pointer[0] = &image[cinfo.next_scanline * row_stride];
jpeg_write_scanlines(&cinfo, row_pointer, 1);
}
jpeg_finish_compress(&cinfo);
fclose(outfile);
jpeg_destroy_compress(&cinfo);
}
```
这个函数接受四个参数:指向unsigned char数组的指针、图像的宽度、高度和保存文件的文件名。它将数组中的数据保存为JPG格式的图像文件。
使用这个函数的示例代码:
```c
int main() {
unsigned char image_data[600][800][3]; // 600x800 RGB image
// ... fill image_data with pixel values ...
save_jpg(&image_data[0][0][0], 800, 600, "image.jpg");
return 0;
}
```
这个示例代码创建了一个600x800的RGB图像数组,并将其保存为名为"image.jpg"的JPG文件。
阅读全文
相关推荐
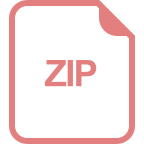
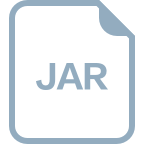
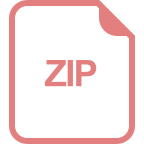

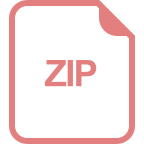
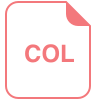



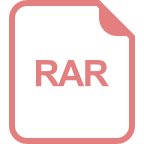
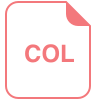
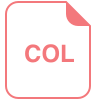
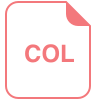






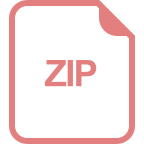