std::find_if
时间: 2023-08-04 11:06:45 浏览: 57
`std::find_if`是C++ STL提供的查找算法,它可以在指定范围内查找满足特定条件的元素,返回第一个符合条件的元素的迭代器。
具体使用方法为:
```c++
template< class InputIt, class UnaryPredicate >
InputIt find_if( InputIt first, InputIt last, UnaryPredicate p );
```
其中:
- `first`和`last`分别是查找范围的起始迭代器和终止迭代器。
- `p`是一个一元谓词函数,它接受一个元素的引用作为参数,返回一个`bool`类型的值。符合条件的元素是指函数返回值为`true`的元素。
- 函数返回第一个符合条件的元素的迭代器。如果没有符合条件的元素,则返回`last`迭代器。
例如,下面的代码使用`std::find_if`在一个整数向量中查找第一个大于等于5的元素:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
int main()
{
std::vector<int> v{1, 3, 5, 7, 9, 2, 4, 6, 8, 10};
auto it = std::find_if(v.begin(), v.end(), [](int x){ return x >= 5; });
if (it != v.end())
std::cout << "Found: " << *it << '\n';
else
std::cout << "Not found\n";
return 0;
}
```
输出结果为:
```
Found: 5
```
相关推荐
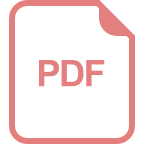
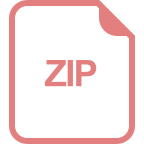














