优化这段代码class Rectangle { int length; int width; Rectangle(int length, int width) { this.length = length; this.width = width; } public int getArea() { return length * width; } public int getGirth() { return (length + width) * 2; } } class Circle { double radius; public static final double PI = 3.14; Circle(double radius) { this.radius = radius; } public double getArea() { return PI * radius * radius; } public double getGirth() { return 2 * PI * radius; } } import java.util.Scanner; public class TestDemo { public static void main(String args[]) { Scanner sc1 = new Scanner(System.in); Scanner sc2 = new Scanner(System.in); { System.out.println("构建矩形并计算其面积和周长"); System.out.println("请输入矩形的长: "); int length = sc1.nextInt(); System.out.println("请输入矩形的宽: "); int width = sc1.nextInt(); Rectangle R1 = new Rectangle(length, width); System.out.println("矩形的面积是: " + R1.getArea()); System.out.println("矩形的周长是: " + R1.getGirth()); System.out.println("构建图形并计算其面积和周长:"); System.out.println("请输入圆形的半径:"); double radius = sc2.nextDouble(); Circle C1 = new Circle(radius); System.out.println("圆形的面积是:" + C1.getArea()); System.out.println("圆形的周长是:" + C1.getGirth()); } } }
时间: 2023-05-24 15:00:44 浏览: 45
这段代码可以通过以下几个优化减少重复代码和提高效率:
1.使用一个 Scanner 对象处理输入,而不是两个不同的对象。
2.在类中定义两个方法,一个计算面积和一个计算周长,而不是每个图形都定义自己的方法。
3.将 PI 定义为常量,而不是每个圆形都定义自己的变量。
修改后的代码如下:
class Rectangle {
int length;
int width;
Rectangle(int length, int width) {
this.length = length;
this.width = width;
}
public int getArea() {
return length * width;
}
public int getGirth() {
return (length + width) * 2;
}
}
class Circle {
double radius;
public static final double PI = 3.14;
Circle(double radius) {
this.radius = radius;
}
public double getArea() {
return PI * radius * radius;
}
public double getGirth() {
return 2 * PI * radius;
}
}
import java.util.Scanner;
public class TestDemo {
public static void main(String args[]) {
Scanner sc = new Scanner(System.in);
System.out.println("构建矩形并计算其面积和周长");
System.out.println("请输入矩形的长: ");
int length = sc.nextInt();
System.out.println("请输入矩形的宽: ");
int width = sc.nextInt();
Rectangle R1 = new Rectangle(length, width);
System.out.println("矩形的面积是: " + R1.getArea());
System.out.println("矩形的周长是: " + R1.getGirth());
System.out.println("构建图形并计算其面积和周长:");
System.out.println("请输入圆形的半径:");
double radius = sc.nextDouble();
Circle C1 = new Circle(radius);
System.out.println("圆形的面积是:" + C1.getArea());
System.out.println("圆形的周长是:" + C1.getGirth());
}
}
相关推荐
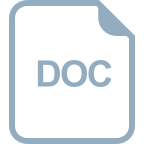
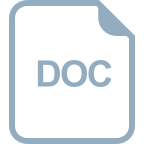
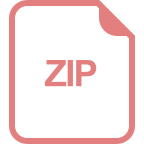















