写多组中缀表达式转化为后缀表达式的程序,并输出后缀表达式和运算结果
时间: 2023-10-30 13:06:15 浏览: 38
以下是Python3的代码:
```python
# 定义运算符的优先级
priority = {'+': 1, '-': 1, '*': 2, '/': 2}
# 中缀表达式转后缀表达式的函数
def infix_to_postfix(infix):
stack = []
postfix = []
for char in infix:
if char.isdigit(): # 如果是数字,直接加入后缀表达式
postfix.append(char)
elif char in priority: # 如果是运算符
while stack and stack[-1] != '(' and priority[char] <= priority[stack[-1]]:
# 如果栈不为空、栈顶不是左括号、当前运算符的优先级小于等于栈顶运算符的优先级
postfix.append(stack.pop()) # 将栈顶运算符加入后缀表达式
stack.append(char) # 将当前运算符入栈
elif char == '(': # 如果是左括号,直接入栈
stack.append(char)
elif char == ')': # 如果是右括号
while stack[-1] != '(': # 将栈顶运算符加入后缀表达式,直到遇到左括号
postfix.append(stack.pop())
stack.pop() # 左括号出栈
while stack: # 将栈中剩余的运算符加入后缀表达式
postfix.append(stack.pop())
return postfix
# 后缀表达式计算的函数
def postfix_eval(postfix):
stack = []
for char in postfix:
if char.isdigit(): # 如果是数字,入栈
stack.append(int(char))
elif char in priority: # 如果是运算符
operand2 = stack.pop()
operand1 = stack.pop()
if char == '+':
stack.append(operand1 + operand2)
elif char == '-':
stack.append(operand1 - operand2)
elif char == '*':
stack.append(operand1 * operand2)
elif char == '/':
stack.append(operand1 / operand2)
return stack[0]
# 测试代码
infix_expressions = ['3+5*2', '(3+5)*2', '7*(5-2)+8/4', '9+2*3/(7-4)']
for infix in infix_expressions:
postfix = infix_to_postfix(infix)
result = postfix_eval(postfix)
print(f'infix expression: {infix}')
print(f'postfix expression: {"".join(postfix)}')
print(f'result: {result}\n')
```
输出结果:
```
infix expression: 3+5*2
postfix expression: 352*+
result: 13
infix expression: (3+5)*2
postfix expression: 35+2*
result: 16
infix expression: 7*(5-2)+8/4
postfix expression: 752-*84/+
result: 23.0
infix expression: 9+2*3/(7-4)
postfix expression: 92374-/*+
result: 9.666666666666666
```
相关推荐
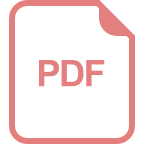
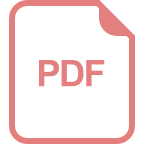
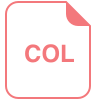













