如何通过txt文档格式,对networkx作为输入,边带权重
时间: 2023-07-18 08:03:50 浏览: 119
可以使用Python中的`read_edgelist`函数从txt文件中读取带权重的边列表。该函数可以将txt文件中的每一行解释为一个边,其中第一列是源节点,第二列是目标节点,第三列是边的权重。
以下是一个示例代码:
```python
import networkx as nx
# 从文件中读取边列表
with open('edges.txt', 'r') as f:
edges = [tuple(map(int, line.strip().split())) for line in f]
# 创建有权图
G = nx.Graph()
# 添加边
for edge in edges:
G.add_edge(edge[0], edge[1], weight=edge[2])
# 打印图的边与权重
for u, v, weight in G.edges.data('weight'):
print(f'({u}, {v}) - {weight}')
```
假设文件`edges.txt`包含以下内容:
```
1 2 3
1 3 4
2 3 1
```
运行上述代码将输出:
```
(1, 2) - 3
(1, 3) - 4
(2, 3) - 1
```
其中每一行表示一条边,第一列是源节点,第二列是目标节点,第三列是边的权重。代码利用`map`函数将每行转换为一个整数元组,并使用`add_edge`方法将带权重的边添加到图中。最后,使用`edges.data`方法打印图的边与权重。
相关问题
networkx对边权重设置以及对图进行加权,代码示范
在networkx中,可以使用`add_weighted_edges_from`方法来添加带权边。示例代码如下:
```python
import networkx as nx
# 创建一个空的有向图
G = nx.DiGraph()
# 添加带权边
G.add_weighted_edges_from([(1, 2, 0.5), (2, 3, 0.75)])
# 输出边权重
for u, v, weight in G.edges.data('weight'):
print(f"({u}, {v}): {weight}")
```
输出结果为:
```
(1, 2): 0.5
(2, 3): 0.75
```
另外,我们也可以在创建图的时候指定边的权重,示例代码如下:
```python
import networkx as nx
# 创建一个空的有向图
G = nx.DiGraph()
# 添加带权边
G.add_edge(1, 2, weight=0.5)
G.add_edge(2, 3, weight=0.75)
# 输出边权重
for u, v, weight in G.edges.data('weight'):
print(f"({u}, {v}): {weight}")
```
输出结果与前面示例相同。
如何用networkx获取加权网络中特定边的权重
你可以使用网络图库networkx中的get_edge_data方法来获取加权网络中特定边的权重。例如,假设你的图形对象为G,特定的节点对为u和v,则可以使用以下命令获取其权重:
G.get_edge_data(u,v)['weight']
相关推荐
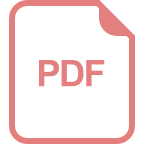
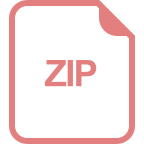












