实现顺序表基本操作算法,建立5个,元素顺序表,在4,5之间插入一个元素9,在删除指定位置i=5上的元素9,输出所有元素,查找任一元素
时间: 2024-10-14 17:07:52 浏览: 6
顺序表是一种线性数据结构,其中元素按照一定的顺序存储在内存的连续区域。以下是实现顺序表的基本操作算法:
1. **创建顺序表**:
- 定义一个数组作为数据结构,初始化长度为0。
```c++
struct ListNode {
int data;
ListNode* next;
};
ListNode* createList() {
ListNode* list = nullptr;
return list;
}
```
2. **插入元素**(在4,5之间插入9):
- 首先检查是否需要扩大列表容量,然后找到插入位置并更新指针。
```c++
void insert(int value, int position, ListNode*& list) {
if (position < 0 || position > size()) {
// 错误处理
return;
}
if (!list) {
list = new ListNode{value, nullptr};
} else {
for (int i = 0; i < position; ++i) {
list = list->next;
}
if (list->next == nullptr) { // 到达末尾
list->next = new ListNode{value, nullptr};
} else {
list->next->prev = list; // 更新前一个节点指向前一个节点
list->next = new ListNode{value, list->next}; // 插入新节点
}
}
}
```
3. **删除元素**(删除位置i=5的元素9):
- 类似于插入,找到目标元素并调整相邻节点的指针。
```c++
void deleteElement(int position, ListNode*& list) {
if (position < 0 || position >= size()) {
return;
}
if (list->next != nullptr && position == 0) {
ListNode* temp = list->next;
list = temp;
delete temp;
} else {
ListNode* current = list;
for (int i = 0; i < position - 1; ++i) {
current = current->next;
}
if (current->next->next != nullptr) {
current->next = current->next->next;
current->next->prev = current;
} else {
// 删除最后一个元素,无需更新 prev 指针
delete current->next;
}
}
}
```
4. **输出所有元素**:
- 使用循环遍历整个列表。
```c++
void printList(ListNode* list) {
ListNode* temp = list;
while (temp != nullptr) {
cout << temp->data << " ";
temp = temp->next;
}
cout << endl;
}
```
5. **查找元素**:
- 遍历列表直到找到目标值或到达末尾。
```c++
bool search(int target, ListNode* list) {
while (list != nullptr) {
if (list->data == target) {
return true;
}
list = list->next;
}
return false;
}
```
相关推荐
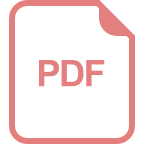
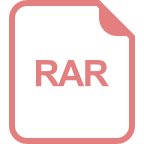
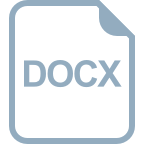















