FunctionalState I2C_WriteByte(uint8_t SendByte, uint16_t WriteAddress, uint8_t DeviceAddress);
时间: 2023-12-01 08:39:34 浏览: 39
根据提供的引用内容,无法确定是否存在函数签名为"FunctionalState I2C_WriteByte(uint8_t SendByte, uint16_t WriteAddress, uint8_t DeviceAddress)"的函数。引用、、中提供了两个函数,一个是进行IIC写操作的函数I2C_WriteByte(uint8_t addr,uint8_t data),另一个是进行IIC读操作的函数I2C_ReadByte(uint8_t addr)。这两个函数的参数和返回值与所提供的函数签名不完全一致。因此,需要更多的信息才能回答这个问题。请提供更多的上下文信息或者代码片段。
相关问题
编写一段STM32 I2C驱动代码
这里提供一个简单的STM32 I2C驱动代码,需要根据具体的芯片型号和硬件连接进行修改。
```c
#include "stm32f4xx.h"
#define I2C_TIMEOUT 10000
void I2C_Init() {
GPIO_InitTypeDef GPIO_InitStruct;
I2C_InitTypeDef I2C_InitStruct;
// Enable I2C clock
RCC_APB1PeriphClockCmd(RCC_APB1Periph_I2C1, ENABLE);
// Enable GPIO clock
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOB, ENABLE);
// Configure I2C pins
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_6 | GPIO_Pin_7;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_AF;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStruct.GPIO_OType = GPIO_OType_OD;
GPIO_InitStruct.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_Init(GPIOB, &GPIO_InitStruct);
// Connect I2C pins to AF
GPIO_PinAFConfig(GPIOB, GPIO_PinSource6, GPIO_AF_I2C1);
GPIO_PinAFConfig(GPIOB, GPIO_PinSource7, GPIO_AF_I2C1);
// Configure I2C
I2C_InitStruct.I2C_ClockSpeed = 100000;
I2C_InitStruct.I2C_Mode = I2C_Mode_I2C;
I2C_InitStruct.I2C_DutyCycle = I2C_DutyCycle_2;
I2C_InitStruct.I2C_OwnAddress1 = 0x00;
I2C_InitStruct.I2C_Ack = I2C_Ack_Enable;
I2C_InitStruct.I2C_AcknowledgedAddress = I2C_AcknowledgedAddress_7bit;
I2C_Init(I2C1, &I2C_InitStruct);
// Enable I2C
I2C_Cmd(I2C1, ENABLE);
}
uint8_t I2C_ReadByte(uint8_t slave_address, uint8_t reg_address) {
uint8_t data = 0;
// Send start condition
I2C_GenerateSTART(I2C1, ENABLE);
while(!I2C_CheckEvent(I2C1, I2C_EVENT_MASTER_MODE_SELECT)) {}
// Send slave address for write
I2C_Send7bitAddress(I2C1, slave_address, I2C_Direction_Transmitter);
while(!I2C_CheckEvent(I2C1, I2C_EVENT_MASTER_TRANSMITTER_MODE_SELECTED)) {}
// Send register address
I2C_SendData(I2C1, reg_address);
while(!I2C_CheckEvent(I2C1, I2C_EVENT_MASTER_BYTE_TRANSMITTED)) {}
// Send repeated start condition
I2C_GenerateSTART(I2C1, ENABLE);
while(!I2C_CheckEvent(I2C1, I2C_EVENT_MASTER_MODE_SELECT)) {}
// Send slave address for read
I2C_Send7bitAddress(I2C1, slave_address, I2C_Direction_Receiver);
while(!I2C_CheckEvent(I2C1, I2C_EVENT_MASTER_RECEIVER_MODE_SELECTED)) {}
// Receive data byte
while(!I2C_CheckEvent(I2C1, I2C_EVENT_MASTER_BYTE_RECEIVED)) {}
data = I2C_ReceiveData(I2C1);
// Send stop condition
I2C_GenerateSTOP(I2C1, ENABLE);
return data;
}
void I2C_WriteByte(uint8_t slave_address, uint8_t reg_address, uint8_t data) {
// Send start condition
I2C_GenerateSTART(I2C1, ENABLE);
while(!I2C_CheckEvent(I2C1, I2C_EVENT_MASTER_MODE_SELECT)) {}
// Send slave address for write
I2C_Send7bitAddress(I2C1, slave_address, I2C_Direction_Transmitter);
while(!I2C_CheckEvent(I2C1, I2C_EVENT_MASTER_TRANSMITTER_MODE_SELECTED)) {}
// Send register address
I2C_SendData(I2C1, reg_address);
while(!I2C_CheckEvent(I2C1, I2C_EVENT_MASTER_BYTE_TRANSMITTED)) {}
// Send data byte
I2C_SendData(I2C1, data);
while(!I2C_CheckEvent(I2C1, I2C_EVENT_MASTER_BYTE_TRANSMITTED)) {}
// Send stop condition
I2C_GenerateSTOP(I2C1, ENABLE);
}
```
这个代码实现了基本的I2C读写操作,使用了STM32的标准库函数。需要注意的是,这个代码只是提供了一个参考,具体的实现还需要根据硬件的具体情况进行调整。
写一段I2C通讯的程序
好的,以下是一个简单的I2C通讯程序示例,使用STM32F103C8T6作为主控芯片,连接一个EEPROM芯片进行读写操作:
```c
#include "stm32f10x.h"
#define I2C_SPEED 100000
#define I2C_EEPROM_ADDR 0xA0
void I2C_Configuration(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
I2C_InitTypeDef I2C_InitStructure;
// Enable GPIOB clock
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE);
// Configure PB6 and PB7 as open-drain output
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_6 | GPIO_Pin_7;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_OD;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOB, &GPIO_InitStructure);
// Enable I2C1 clock
RCC_APB1PeriphClockCmd(RCC_APB1Periph_I2C1, ENABLE);
// Configure I2C1 parameters
I2C_InitStructure.I2C_Mode = I2C_Mode_I2C;
I2C_InitStructure.I2C_DutyCycle = I2C_DutyCycle_2;
I2C_InitStructure.I2C_OwnAddress1 = 0;
I2C_InitStructure.I2C_Ack = I2C_Ack_Enable;
I2C_InitStructure.I2C_AcknowledgedAddress = I2C_AcknowledgedAddress_7bit;
I2C_InitStructure.I2C_ClockSpeed = I2C_SPEED;
I2C_Init(I2C1, &I2C_InitStructure);
// Enable I2C1
I2C_Cmd(I2C1, ENABLE);
}
void I2C_EEPROM_WriteByte(uint16_t WriteAddr, uint8_t Data)
{
uint8_t TxBuffer[2];
// Build Tx buffer
TxBuffer[0] = WriteAddr >> 8;
TxBuffer[1] = WriteAddr & 0xFF;
TxBuffer[2] = Data;
// Send start condition
I2C_GenerateSTART(I2C1, ENABLE);
// Wait until start condition is sent
while (!I2C_CheckEvent(I2C1, I2C_EVENT_MASTER_MODE_SELECT));
// Send EEPROM address (write mode)
I2C_Send7bitAddress(I2C1, I2C_EEPROM_ADDR, I2C_Direction_Transmitter);
// Wait until EEPROM address is sent
while (!I2C_CheckEvent(I2C1, I2C_EVENT_MASTER_TRANSMITTER_MODE_SELECTED));
// Send data
I2C_SendData(I2C1, TxBuffer[0]);
// Wait until data is sent
while (!I2C_CheckEvent(I2C1, I2C_EVENT_MASTER_BYTE_TRANSMITTED));
I2C_SendData(I2C1, TxBuffer[1]);
while (!I2C_CheckEvent(I2C1, I2C_EVENT_MASTER_BYTE_TRANSMITTED));
I2C_SendData(I2C1, TxBuffer[2]);
while (!I2C_CheckEvent(I2C1, I2C_EVENT_MASTER_BYTE_TRANSMITTED));
// Send stop condition
I2C_GenerateSTOP(I2C1, ENABLE);
}
uint8_t I2C_EEPROM_ReadByte(uint16_t ReadAddr)
{
uint8_t RxBuffer[2];
// Build Tx buffer
TxBuffer[0] = ReadAddr >> 8;
TxBuffer[1] = ReadAddr & 0xFF;
// Send start condition
I2C_GenerateSTART(I2C1, ENABLE);
// Wait until start condition is sent
while (!I2C_CheckEvent(I2C1, I2C_EVENT_MASTER_MODE_SELECT));
// Send EEPROM address (write mode)
I2C_Send7bitAddress(I2C1, I2C_EEPROM_ADDR, I2C_Direction_Transmitter);
// Wait until EEPROM address is sent
while (!I2C_CheckEvent(I2C1, I2C_EVENT_MASTER_TRANSMITTER_MODE_SELECTED));
// Send data
I2C_SendData(I2C1, TxBuffer[0]);
// Wait until data is sent
while (!I2C_CheckEvent(I2C1, I2C_EVENT_MASTER_BYTE_TRANSMITTED));
I2C_SendData(I2C1, TxBuffer[1]);
while (!I2C_CheckEvent(I2C1, I2C_EVENT_MASTER_BYTE_TRANSMITTED));
// Send repeated start condition
I2C_GenerateSTART(I2C1, ENABLE);
// Wait until repeated start condition is sent
while (!I2C_CheckEvent(I2C1, I2C_EVENT_MASTER_MODE_SELECT));
// Send EEPROM address (read mode)
I2C_Send7bitAddress(I2C1, I2C_EEPROM_ADDR, I2C_Direction_Receiver);
// Wait until EEPROM address is sent
while (!I2C_CheckEvent(I2C1, I2C_EVENT_MASTER_RECEIVER_MODE_SELECTED));
// Disable ACK
I2C_AcknowledgeConfig(I2C1, DISABLE);
// Send stop condition
I2C_GenerateSTOP(I2C1, ENABLE);
// Wait until data is received
while (!I2C_CheckEvent(I2C1, I2C_EVENT_MASTER_BYTE_RECEIVED));
// Read data
RxBuffer[0] = I2C_ReceiveData(I2C1);
// Wait until data is received
while (!I2C_CheckEvent(I2C1, I2C_EVENT_MASTER_BYTE_RECEIVED));
RxBuffer[1] = I2C_ReceiveData(I2C1);
// Wait until stop condition is detected
while (!I2C_CheckEvent(I2C1, I2C_EVENT_MASTER_STOP_DETECTED));
// Enable ACK
I2C_AcknowledgeConfig(I2C1, ENABLE);
return RxBuffer[1];
}
int main(void)
{
// Configure I2C
I2C_Configuration();
// Write data to EEPROM
I2C_EEPROM_WriteByte(0x0000, 0xAA);
// Read data from EEPROM
uint8_t data = I2C_EEPROM_ReadByte(0x0000);
while (1);
}
```
以上代码仅供参考,具体实现需要根据实际情况进行调整和优化。
相关推荐
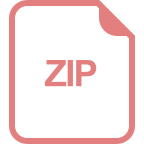
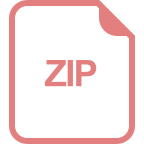










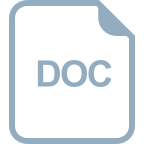