c++复数类>>重载具体代码怎么写
时间: 2024-10-21 11:06:51 浏览: 24
在 C++ 中,创建复数类并实现加法、减法等运算通常会涉及到复数运算的特殊规则。首先,我们需要定义一个名为 `Complex` 的类,包含实部(`real`)和虚部(`imaginary`)。然后,我们可以为这个类提供一些成员函数,如构造函数、赋值操作符和运算符重载。
以下是简单的复数类及部分运算符重载的代码示例:
```cpp
#include <iostream>
class Complex {
public:
// 构造函数
Complex(double r = 0.0, double i = 0.0) : real(r), imaginary(i) {}
// 数据成员
double real;
double imaginary;
// 赋值操作符重载
Complex& operator=(const Complex& other) {
if (this != &other) { // 防止自我赋值
real = other.real;
imaginary = other.imaginary;
}
return *this;
}
// 加法运算符重载
Complex operator+(const Complex& other) const {
return Complex(real + other.real, imaginary + other.imaginary);
}
// 减法运算符重载
Complex operator-(const Complex& other) const {
return Complex(real - other.real, imaginary - other.imaginary);
}
// 另外还可以考虑除法、乘法等运算符重载
// 输出复数
friend std::ostream& operator<<(std::ostream& os, const Complex& complex) {
return os << complex.real << " + " << complex.imaginary << "i";
}
};
int main() {
Complex c1(3.0, 4.0);
Complex c2(1.0, -2.0);
Complex sum = c1 + c2; // 自动调用加法运算符
std::cout << "c1 + c2 = " << sum << std::endl;
return 0;
}
```
在这个例子中,我们重载了 `+` 和 `-` 运算符,使得可以对两个复数对象进行相加和相减的操作。注意,为了能够输出复数结果,我们还添加了一个友元函数 `operator<<` 来配合 `std::cout`。
阅读全文
相关推荐
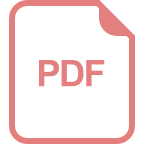
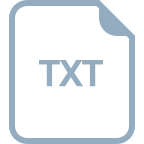
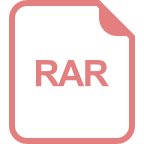

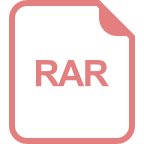
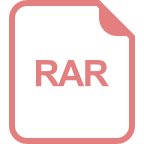
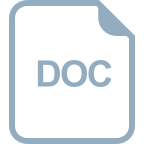
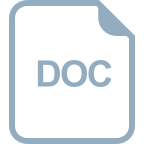
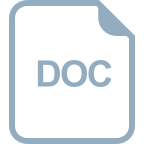
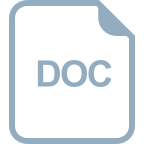
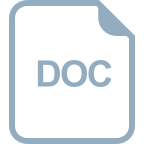
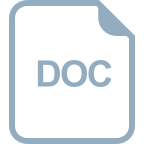






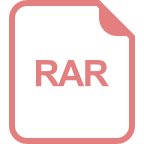