如何使用Switch调用同一基类里的不同派生类函数C++
时间: 2024-09-11 09:17:35 浏览: 47
在C++中,使用switch语句调用同一基类的不同派生类函数通常不是直接实现的,因为switch语句是基于整数或枚举类型进行选择的,而不是基于类类型的。但是,我们可以通过一些间接的方式实现类似的功能,比如将派生类与枚举类型或整数相关联,然后在switch语句中使用这些枚举或整数来执行不同的操作。
以下是一个简单的例子,演示如何使用枚举类型和switch语句来根据不同的情况调用基类指针所指向的派生类对象的函数。
```cpp
#include <iostream>
// 基类
class Base {
public:
virtual void doSomething() const {
std::cout << "Base class function called." << std::endl;
}
};
// 派生类1
class Derived1 : public Base {
public:
void doSomething() const override {
std::cout << "Derived1 class function called." << std::endl;
}
};
// 派生类2
class Derived2 : public Base {
public:
void doSomething() const override {
std::cout << "Derived2 class function called." << std::endl;
}
};
int main() {
// 使用枚举来表示不同的派生类
enum class DerivedType { Derived1, Derived2 };
Base* basePtr;
DerivedType type = DerivedType::Derived1; // 假设这是根据某些条件确定的类型
switch(type) {
case DerivedType::Derived1:
basePtr = new Derived1();
break;
case DerivedType::Derived2:
basePtr = new Derived2();
break;
default:
basePtr = nullptr;
break;
}
if(basePtr) {
basePtr->doSomething(); // 这里会调用派生类的doSomething函数
delete basePtr; // 记得释放内存
}
return 0;
}
```
在上面的代码中,我们定义了一个枚举类型`DerivedType`来区分不同的派生类。在`main`函数中,我们根据`type`的值,使用`new`关键字创建相应的派生类对象,并将其赋值给基类指针`basePtr`。然后通过`switch`语句选择不同的处理路径,最后通过基类指针调用`doSomething`函数。
需要注意的是,这种方法需要在使用`switch`之前就明确知道将要创建的是哪个派生类的对象,并且在调用`doSomething`时,多态的特性会保证调用正确的方法。
阅读全文
相关推荐
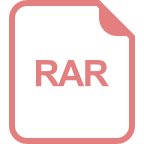
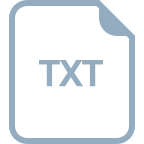
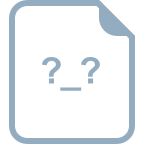















